Cloud
How do I use Cloud
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: GUICustomAuth.cs
Copy
81 void OnGUI()
82 {
83 if (PhotonNetwork.connected)
84 {
85 GUILayout.Label(PhotonNetwork.connectionStateDetailed.ToString());
86 return;
87 }
88
89
90 GUILayout.BeginArea(GuiRect);
91 switch (guiState)
92 {
93 case GuiState.AuthFailed:
94 GUILayout.Label("Authentication Failed");
95
96 GUILayout.Space(10);
97
98 GUILayout.Label("Error message:\n'" + this.authDebugMessage + "'");
99
100 GUILayout.Space(10);
101
102 GUILayout.Label("For this demo set the Authentication URL in the Dashboard to:\nhttp://photon.webscript.io/auth-demo-equals");
103 GUILayout.Label("That authentication-service has no user-database. It confirms any user if 'name equals password'.");
104 GUILayout.Label("The error message comes from that service and can be customized.");
105
106 GUILayout.Space(10);
107
108 GUILayout.BeginHorizontal();
109 if (GUILayout.Button("Back"))
110 {
111 SetStateAuthInput();
112 }
113 if (GUILayout.Button("Help"))
114 {
115 SetStateAuthHelp();
116 }
117 GUILayout.EndHorizontal();
118 break;
119
120 case GuiState.AuthHelp:
121
122 GUILayout.Label("By default, any player can connect to Photon.\n'Custom Authentication' can be enabled to reject players without valid user-account.");
123
124 GUILayout.Label("The actual authentication must be done by a web-service which you host and customize. Example sourcecode for these services is available on the docs page.");
125
126 GUILayout.Label("For this demo set the Authentication URL in the Dashboard to:\nhttp://photon.webscript.io/auth-demo-equals");
127 GUILayout.Label("That authentication-service has no user-database. It confirms any user if 'name equals password'.");
128
129 GUILayout.Space(10);
130 if (GUILayout.Button("Configure Authentication (Dashboard)"))
131 {
132 Application.OpenURL("https://cloud.exitgames.com/dashboard");
133 }
134 if (GUILayout.Button("Authentication Docs"))
135 {
136 Application.OpenURL("https://doc.exitgames.com/en/pun/current/tutorials/pun-and-facebook-custom-authentication");
137 }
138
139
140 GUILayout.Space(10);
141 if (GUILayout.Button("Back to input"))
142 {
143 SetStateAuthInput();
144 }
145 break;
146
147 case GuiState.AuthInput:
148
149 GUILayout.Label("Authenticate yourself");
150
151 GUILayout.BeginHorizontal();
152 this.authName = GUILayout.TextField(this.authName, GUILayout.Width(Screen.width/4 - 5));
153 GUILayout.FlexibleSpace();
154 this.authToken = GUILayout.TextField(this.authToken, GUILayout.Width(Screen.width/4 - 5));
155 GUILayout.EndHorizontal();
156
157
158 if (GUILayout.Button("Authenticate"))
159 {
160 PhotonNetwork.AuthValues = new AuthenticationValues();
161 PhotonNetwork.AuthValues.SetAuthParameters(this.authName, this.authToken);
162 PhotonNetwork.ConnectUsingSettings("1.0");
163 }
164
165 GUILayout.Space(10);
166
167 if (GUILayout.Button("Help", GUILayout.Width(100)))
168 {
169 SetStateAuthHelp();
170 }
171
172 break;
173 }
174
175 GUILayout.EndArea();
176 }
File name: AccountService.cs
Copy
37 public enum Origin : byte { ServerWeb = 1, CloudWeb = 2, Pun = 3, Playmaker = 4 };
File name: AccountService.cs
Copy
59 public void RegisterByEmail(string email, Origin origin)
60 {
61 this.registrationCallback = null;
62 this.AppId = string.Empty;
63 this.Message = string.Empty;
64 this.ReturnCode = -1;
65
66 string result;
67 try
68 {
69 WebRequest req = HttpWebRequest.Create(this.RegistrationUri(email, (byte)origin));
70 HttpWebResponse resp = req.GetResponse() as HttpWebResponse;
71
72 // now read result
73 StreamReader reader = new StreamReader(resp.GetResponseStream());
74 result = reader.ReadToEnd();
75 }
76 catch (Exception ex)
77 {
78 this.Message = "Failed to connect to Cloud Account Service. Please register via account website.";
79 this.Exception = ex;
80 return;
81 }
82
83 this.ParseResult(result);
84 }
File name: AccountService.cs
Copy
93 public void RegisterByEmailAsync(string email, Origin origin, Action94 {
95 this.registrationCallback = callback;
96 this.AppId = string.Empty;
97 this.Message = string.Empty;
98 this.ReturnCode = -1;
99
100 try
101 {
102 HttpWebRequest req = (HttpWebRequest)HttpWebRequest.Create(this.RegistrationUri(email, (byte)origin));
103 req.Timeout = 5000;
104 req.BeginGetResponse(this.OnRegisterByEmailCompleted, req);
105 }
106 catch (Exception ex)
107 {
108 this.Message = "Failed to connect to Cloud Account Service. Please register via account website.";
109 this.Exception = ex;
110 if (this.registrationCallback != null)
111 {
112 this.registrationCallback(this);
113 }
114 }
115 }
File name: AccountService.cs
Copy
121 private void OnRegisterByEmailCompleted(IAsyncResult ar)
122 {
123 try
124 {
125 HttpWebRequest request = (HttpWebRequest)ar.AsyncState;
126 HttpWebResponse response = request.EndGetResponse(ar) as HttpWebResponse;
127
128 if (response != null && response.StatusCode == HttpStatusCode.OK)
129 {
130 // no error. use the result
131 StreamReader reader = new StreamReader(response.GetResponseStream());
132 string result = reader.ReadToEnd();
133
134 this.ParseResult(result);
135 }
136 else
137 {
138 // a response but some error on server. show message
139 this.Message = "Failed to connect to Cloud Account Service. Please register via account website.";
140 }
141 }
142 catch (Exception ex)
143 {
144 // not even a response. show message
145 this.Message = "Failed to connect to Cloud Account Service. Please register via account website.";
146 this.Exception = ex;
147 }
148
149 if (this.registrationCallback != null)
150 {
151 this.registrationCallback(this);
152 }
153 }
File name: PhotonEditor.cs
Copy
147 {
148 RegisterForPhotonCloud,
149
150 EmailAlreadyRegistered,
151
152 SetupPhotonCloud,
153
154 SetupSelfHosted
155 }
File name: PhotonEditor.cs
Copy
215 static PhotonEditor()
216 {
217 EditorApplication.projectWindowChanged += EditorUpdate;
218 EditorApplication.hierarchyWindowChanged += EditorUpdate;
219 EditorApplication.playmodeStateChanged += PlaymodeStateChanged;
220 EditorApplication.update += OnUpdate;
221
222 WizardIcon = AssetDatabase.LoadAssetAtPath("Assets/Photon Unity Networking/photoncloud-icon.png", typeof(Texture2D)) as Texture2D;
223
224 // to be used in toolbar, the enum needs conversion to string[] being done here, once.
225 Array enumValues = Enum.GetValues(typeof(CloudRegionCode));
226 CloudServerRegionNames = new string[enumValues.Length];
227 for (int i = 0; i < CloudServerRegionNames.Length; i++)
228 {
229 CloudServerRegionNames[i] = enumValues.GetValue(i).ToString();
230 if (CloudServerRegionNames[i].Equals("none"))
231 {
232 CloudServerRegionNames[i] = PhotonEditor.CurrentLang.BestRegionLabel;
233 }
234 }
235
236 // detect optional packages
237 PhotonEditor.CheckPunPlus();
238
239 }
File name: PhotonEditor.cs
Copy
294 protected void InitPhotonSetupWindow()
295 {
296 this.minSize = MinSize;
297
298 this.SwitchMenuState(GUIState.Setup);
299 this.ReApplySettingsToWindow();
300
301 switch (PhotonEditor.Current.HostType)
302 {
303 case ServerSettings.HostingOption.PhotonCloud:
304 case ServerSettings.HostingOption.BestRegion:
305 this.photonSetupState = PhotonSetupStates.SetupPhotonCloud;
306 break;
307 case ServerSettings.HostingOption.SelfHosted:
308 this.photonSetupState = PhotonSetupStates.SetupSelfHosted;
309 break;
310 case ServerSettings.HostingOption.NotSet:
311 default:
312 this.photonSetupState = PhotonSetupStates.RegisterForPhotonCloud;
313 break;
314 }
315 }
File name: PhotonEditor.cs
Copy
390 protected virtual void OnGUI()
391 {
392 PhotonSetupStates oldGuiState = this.photonSetupState; // used to fix an annoying Editor input field issue: wont refresh until focus is changed.
393
394 GUI.SetNextControlName("");
395 this.scrollPos = GUILayout.BeginScrollView(this.scrollPos);
396
397 if (this.guiState == GUIState.Uninitialized)
398 {
399 this.ReApplySettingsToWindow();
400 this.guiState = (PhotonEditor.Current.HostType == ServerSettings.HostingOption.NotSet) ? GUIState.Setup : GUIState.Main;
401 }
402
403 if (this.guiState == GUIState.Main)
404 {
405 this.OnGuiMainWizard();
406 }
407 else
408 {
409 this.OnGuiRegisterCloudApp();
410 }
411
412 GUILayout.EndScrollView();
413
414 if (oldGuiState != this.photonSetupState)
415 {
416 GUI.FocusControl("");
417 }
418 }
File name: PhotonEditor.cs
Copy
420 protected virtual void OnGuiRegisterCloudApp()
421 {
422 GUI.skin.label.wordWrap = true;
423 if (!this.isSetupWizard)
424 {
425 GUILayout.BeginHorizontal();
426 GUILayout.FlexibleSpace();
427 if (GUILayout.Button(CurrentLang.MainMenuButton, GUILayout.ExpandWidth(false)))
428 {
429 this.SwitchMenuState(GUIState.Main);
430 }
431
432 GUILayout.EndHorizontal();
433
434 GUILayout.Space(15);
435 }
436
437 if (this.photonSetupState == PhotonSetupStates.RegisterForPhotonCloud)
438 {
439 GUI.skin.label.fontStyle = FontStyle.Bold;
440 GUILayout.Label(CurrentLang.ConnectButton);
441 EditorGUILayout.Separator();
442 GUI.skin.label.fontStyle = FontStyle.Normal;
443
444 GUILayout.Label(CurrentLang.UsePhotonLabel);
445 EditorGUILayout.Separator();
446 this.emailAddress = EditorGUILayout.TextField(CurrentLang.EmailLabel, this.emailAddress);
447
448 if (GUILayout.Button(CurrentLang.SendButton))
449 {
450 GUIUtility.keyboardControl = 0;
451 this.RegisterWithEmail(this.emailAddress);
452 }
453
454 GUILayout.Space(20);
455
456
457 GUILayout.Label(CurrentLang.SignedUpAlreadyLabel);
458 if (GUILayout.Button(CurrentLang.SetupButton))
459 {
460 this.photonSetupState = PhotonSetupStates.SetupPhotonCloud;
461 }
462 EditorGUILayout.Separator();
463
464
465 GUILayout.Label(CurrentLang.RegisterByWebsiteLabel);
466 if (GUILayout.Button(CurrentLang.AccountWebsiteButton))
467 {
468 EditorUtility.OpenWithDefaultApp(UrlAccountPage + Uri.EscapeUriString(this.emailAddress));
469 }
470
471 EditorGUILayout.Separator();
472
473 GUILayout.Label(CurrentLang.SelfHostLabel);
474
475 if (GUILayout.Button(CurrentLang.SelfHostSettingsButton))
476 {
477 this.photonSetupState = PhotonSetupStates.SetupSelfHosted;
478 }
479
480 GUILayout.FlexibleSpace();
481
482
483 if (!InternalEditorUtility.HasAdvancedLicenseOnBuildTarget(BuildTarget.Android) || !InternalEditorUtility.HasAdvancedLicenseOnBuildTarget(BuildTarget.iOS))
484 {
485 GUILayout.Label(CurrentLang.MobileExportNoteLabel);
486 }
487 EditorGUILayout.Separator();
488 }
489 else if (this.photonSetupState == PhotonSetupStates.EmailAlreadyRegistered)
490 {
491 GUI.skin.label.fontStyle = FontStyle.Bold;
492 GUILayout.Label(CurrentLang.OopsLabel);
493 GUI.skin.label.fontStyle = FontStyle.Normal;
494
495 GUILayout.Label(CurrentLang.EmailInUseLabel);
496
497 if (GUILayout.Button(CurrentLang.SeeMyAccountPageButton))
498 {
499 EditorUtility.OpenWithDefaultApp(UrlCloudDashboard + Uri.EscapeUriString(this.emailAddress));
500 }
501
502 EditorGUILayout.Separator();
503
504 GUILayout.Label(CurrentLang.KnownAppIdLabel);
505 GUILayout.BeginHorizontal();
506 if (GUILayout.Button(CurrentLang.CancelButton))
507 {
508 this.photonSetupState = PhotonSetupStates.RegisterForPhotonCloud;
509 }
510
511 if (GUILayout.Button(CurrentLang.SetupButton))
512 {
513 this.photonSetupState = PhotonSetupStates.SetupPhotonCloud;
514 }
515
516 GUILayout.EndHorizontal();
517 }
518 else if (this.photonSetupState == PhotonSetupStates.SetupPhotonCloud)
519 {
520 // cloud setup
521 GUI.skin.label.fontStyle = FontStyle.Bold;
522 GUILayout.Label(CurrentLang.PhotonCloudConnect);
523 GUI.skin.label.fontStyle = FontStyle.Normal;
524
525 EditorGUILayout.Separator();
526 this.OnGuiSetupCloudAppId();
527 this.OnGuiCompareAndHelpOptions();
528 }
529 else if (this.photonSetupState == PhotonSetupStates.SetupSelfHosted)
530 {
531 // self-hosting setup
532 GUI.skin.label.fontStyle = FontStyle.Bold;
533 GUILayout.Label(CurrentLang.SetupOwnHostLabel);
534 GUI.skin.label.fontStyle = FontStyle.Normal;
535
536 EditorGUILayout.Separator();
537
538 this.OnGuiSetupSelfhosting();
539 this.OnGuiCompareAndHelpOptions();
540 }
541 }
Download file with original file name:Cloud
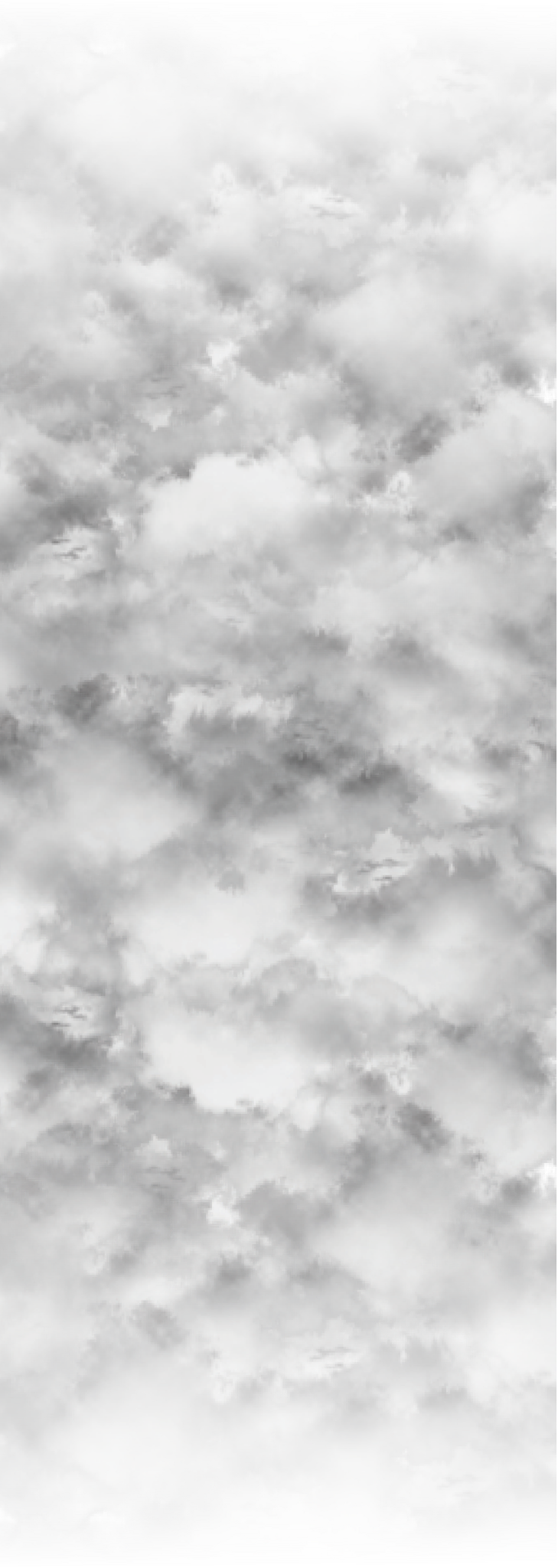
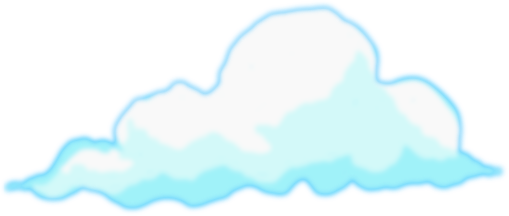
Cloud 98 lượt xem
Gõ tìm kiếm nhanh...