Done
How do I use Done
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: GUICustomAuth.cs
Copy
81 void OnGUI()
82 {
83 if (PhotonNetwork.connected)
84 {
85 GUILayout.Label(PhotonNetwork.connectionStateDetailed.ToString());
86 return;
87 }
88
89
90 GUILayout.BeginArea(GuiRect);
91 switch (guiState)
92 {
93 case GuiState.AuthFailed:
94 GUILayout.Label("Authentication Failed");
95
96 GUILayout.Space(10);
97
98 GUILayout.Label("Error message:\n'" + this.authDebugMessage + "'");
99
100 GUILayout.Space(10);
101
102 GUILayout.Label("For this demo set the Authentication URL in the Dashboard to:\nhttp://photon.webscript.io/auth-demo-equals");
103 GUILayout.Label("That authentication-service has no user-database. It confirms any user if 'name equals password'.");
104 GUILayout.Label("The error message comes from that service and can be customized.");
105
106 GUILayout.Space(10);
107
108 GUILayout.BeginHorizontal();
109 if (GUILayout.Button("Back"))
110 {
111 SetStateAuthInput();
112 }
113 if (GUILayout.Button("Help"))
114 {
115 SetStateAuthHelp();
116 }
117 GUILayout.EndHorizontal();
118 break;
119
120 case GuiState.AuthHelp:
121
122 GUILayout.Label("By default, any player can connect to Photon.\n'Custom Authentication' can be enabled to reject players without valid user-account.");
123
124 GUILayout.Label("The actual authentication must be done by a web-service which you host and customize. Example sourcecode for these services is available on the docs page.");
125
126 GUILayout.Label("For this demo set the Authentication URL in the Dashboard to:\nhttp://photon.webscript.io/auth-demo-equals");
127 GUILayout.Label("That authentication-service has no user-database. It confirms any user if 'name equals password'.");
128
129 GUILayout.Space(10);
130 if (GUILayout.Button("Configure Authentication (Dashboard)"))
131 {
132 Application.OpenURL("https://cloud.exitgames.com/dashboard");
133 }
134 if (GUILayout.Button("Authentication Docs"))
135 {
136 Application.OpenURL("https://doc.exitgames.com/en/pun/current/tutorials/pun-and-facebook-custom-authentication");
137 }
138
139
140 GUILayout.Space(10);
141 if (GUILayout.Button("Back to input"))
142 {
143 SetStateAuthInput();
144 }
145 break;
146
147 case GuiState.AuthInput:
148
149 GUILayout.Label("Authenticate yourself");
150
151 GUILayout.BeginHorizontal();
152 this.authName = GUILayout.TextField(this.authName, GUILayout.Width(Screen.width/4 - 5));
153 GUILayout.FlexibleSpace();
154 this.authToken = GUILayout.TextField(this.authToken, GUILayout.Width(Screen.width/4 - 5));
155 GUILayout.EndHorizontal();
156
157
158 if (GUILayout.Button("Authenticate"))
159 {
160 PhotonNetwork.AuthValues = new AuthenticationValues();
161 PhotonNetwork.AuthValues.SetAuthParameters(this.authName, this.authToken);
162 PhotonNetwork.ConnectUsingSettings("1.0");
163 }
164
165 GUILayout.Space(10);
166
167 if (GUILayout.Button("Help", GUILayout.Width(100)))
168 {
169 SetStateAuthHelp();
170 }
171
172 break;
173 }
174
175 GUILayout.EndArea();
176 }
File name: PunStartup.cs
Copy
25 static PunStartup()
26 {
27 bool doneBefore = EditorPrefs.GetBool("PunDemosOpenedBefore");
28 if (!doneBefore)
29 {
30 EditorApplication.update += OnUpdate;
31 }
32 }
File name: PunStartup.cs
Copy
34 static void OnUpdate()
35 {
36 bool doneBefore = EditorPrefs.GetBool("PunDemosOpenedBefore");
37 if (doneBefore)
38 {
39 EditorApplication.update -= OnUpdate;
40 return;
41 }
42
43 if (String.IsNullOrEmpty(EditorApplication.currentScene) && EditorBuildSettings.scenes.Length == 0)
44 {
45 #if UNITY_4_2 || UNITY_4_3 || UNITY_4_4 || UNITY_4_5 || UNITY_4_6 || UNITY_5 || UNITY_5_1 || UNITY_5_2
46 if (EditorApplication.isUpdating) return;
47 #endif
48
49 LoadPunDemoHub();
50 SetPunDemoBuildSettings();
51 EditorPrefs.SetBool("PunDemosOpenedBefore", true);
52 Debug.Log("No scene was open. Loaded PUN Demo Hub Scene and added demos to build settings. Ready to go! This auto-setup is now disabled in this Editor.");
53 }
54 }
File name: PhotonConverter.cs
Copy
120 public static void PickFolderAndConvertScripts()
121 {
122 string folderPath = EditorUtility.OpenFolderPanel("Pick source folder to convert", Directory.GetCurrentDirectory(), "");
123 if (string.IsNullOrEmpty(folderPath))
124 {
125 EditorUtility.DisplayDialog("Script Conversion", "No folder was selected. No files were changed. Please start over.", "Ok.");
126 return;
127 }
128
129 bool result = EditorUtility.DisplayDialog("Script Conversion", "Scripts in this folder will be modified:\n\n" + folderPath + "\n\nMake sure you have backups of these scripts.\nConversion is not guaranteed to work!", "Backup done. Go!", "Abort");
130 if (!result)
131 {
132 return;
133 }
134
135 List
136 ConvertScripts(scripts);
137
138 EditorUtility.DisplayDialog("Script Conversion", "Scripts are now converted to PUN.\n\nYou will need to update\n- scenes\n- components\n- prefabs and\n- add \"PhotonNetwork.ConnectWithDefaultSettings();\"", "Ok");
139 }
File name: PhotonEditor.cs
Copy
318 private static void OnUpdate()
319 {
320 // after a compile, check RPCs to create a cache-list
321 if (!postCompileActionsDone && !EditorApplication.isCompiling && !EditorApplication.isPlayingOrWillChangePlaymode && PhotonEditor.Current != null)
322 {
323 #if UNITY_4_2 || UNITY_4_3 || UNITY_4_4 || UNITY_4_5 || UNITY_4_6 || UNITY_5 || UNITY_5_1 || UNITY_5_2
324 if (EditorApplication.isUpdating) return;
325 #endif
326
327 PhotonEditor.UpdateRpcList();
328 postCompileActionsDone = true; // on compile, this falls back to false (without actively doing anything)
329
330 #if UNITY_4_2 || UNITY_4_3 || UNITY_4_4 || UNITY_4_5 || UNITY_4_6 || UNITY_5 || UNITY_5_1 || UNITY_5_2
331 PhotonEditor.ImportWin8Support();
332 #endif
333 }
334 }
File name: PhotonViewHandler.cs
Copy
25 internal static void HierarchyChange()
26 {
27 if (Application.isPlaying)
28 {
29 //Debug.Log("HierarchyChange ignored, while running.");
30 CheckSceneForStuckHandlers = true; // done once AFTER play mode.
31 return;
32 }
33
34 if (CheckSceneForStuckHandlers)
35 {
36 CheckSceneForStuckHandlers = false;
37 PhotonNetwork.InternalCleanPhotonMonoFromSceneIfStuck();
38 }
39
40 HashSet
41 HashSet
42 bool fixedSomeId = false;
43
44 //// the following code would be an option if we only checked scene objects (but we can check all PVs)
45 //PhotonView[] pvObjects = GameObject.FindSceneObjectsOfType(typeof(PhotonView)) as PhotonView[];
46 //Debug.Log("HierarchyChange. PV Count: " + pvObjects.Length);
47
48 string levelName = Application.loadedLevelName;
49 #if UNITY_EDITOR
50 levelName = System.IO.Path.GetFileNameWithoutExtension(EditorApplication.currentScene);
51 #endif
52 int minViewIdInThisScene = PunSceneSettings.MinViewIdForScene(levelName);
53 //Debug.Log("Level '" + Application.loadedLevelName + "' has a minimum ViewId of: " + minViewIdInThisScene);
54
55 PhotonView[] pvObjects = Resources.FindObjectsOfTypeAll(typeof(PhotonView)) as PhotonView[];
56
57 foreach (PhotonView view in pvObjects)
58 {
59 // first pass: fix prefabs to viewID 0 if they got a view number assigned (cause they should not have one!)
60 if (EditorUtility.IsPersistent(view.gameObject))
61 {
62 if (view.viewID != 0 || view.prefixBackup != -1 || view.instantiationId != -1)
63 {
64 Debug.LogWarning("PhotonView on persistent object being fixed (id and prefix must be 0). Was: " + view);
65 view.viewID = 0;
66 view.prefixBackup = -1;
67 view.instantiationId = -1;
68 EditorUtility.SetDirty(view);
69 fixedSomeId = true;
70 }
71 }
72 else
73 {
74 // keep all scene-instanced PVs for later re-check
75 pvInstances.Add(view);
76 }
77 }
78
79 Dictionary
80
81 // second pass: check all used-in-scene viewIDs for duplicate viewIDs (only checking anything non-prefab)
82 // scene-PVs must have user == 0 (scene/room) and a subId != 0
83 foreach (PhotonView view in pvInstances)
84 {
85 if (view.ownerId > 0)
86 {
87 Debug.Log("Re-Setting Owner ID of: " + view);
88 }
89 view.ownerId = 0; // simply make sure no owner is set (cause room always uses 0)
90 view.prefix = -1; // TODO: prefix could be settable via inspector per scene?!
91
92 if (view.viewID != 0)
93 {
94 if (view.viewID < minViewIdInThisScene || usedInstanceViewNumbers.Contains(view.viewID))
95 {
96 view.viewID = 0; // avoid duplicates and negative values by assigning 0 as (temporary) number to be fixed in next pass
97 }
98 else
99 {
100 usedInstanceViewNumbers.Add(view.viewID); // builds a list of currently used viewIDs
101
102 int instId = 0;
103 if (idPerObject.TryGetValue(view.gameObject, out instId))
104 {
105 view.instantiationId = instId;
106 }
107 else
108 {
109 view.instantiationId = view.viewID;
110 idPerObject[view.gameObject] = view.instantiationId;
111 }
112 }
113 }
114
115 }
116
117 // third pass: anything that's now 0 must get a new (not yet used) ID (starting at 0)
118 int lastUsedId = (minViewIdInThisScene > 0) ? minViewIdInThisScene - 1 : 0;
119
120 foreach (PhotonView view in pvInstances)
121 {
122 if (view.viewID == 0)
123 {
124 // Debug.LogWarning("setting scene ID: " + view.gameObject.name + " ID: " + view.subId.ID + " scene ID: " + view.GetSceneID() + " IsPersistent: " + EditorUtility.IsPersistent(view.gameObject) + " IsSceneViewIDFree: " + IsSceneViewIDFree(view.subId.ID, view));
125 int nextViewId = PhotonViewHandler.GetID(lastUsedId, usedInstanceViewNumbers);
126
127 view.viewID = nextViewId;
128
129 int instId = 0;
130 if (idPerObject.TryGetValue(view.gameObject, out instId))
131 {
132 Debug.Log("Set inst ID");
133 view.instantiationId = instId;
134 }
135 else
136 {
137 view.instantiationId = view.viewID;
138 idPerObject[view.gameObject] = nextViewId;
139 }
140
141 //// when using the Editor's serialization (view.subId in this case), this is not needed, it seems
142 //PrefabUtility.RecordPrefabInstancePropertyModifications(view);
143
144 lastUsedId = nextViewId;
145 EditorUtility.SetDirty(view);
146 fixedSomeId = true;
147 }
148 }
149
150
151 if (fixedSomeId)
152 {
153 //Debug.LogWarning("Some subId was adjusted."); // this log is only interesting for Exit Games
154 }
155 }
File name: NetworkingPeer.cs
Copy
757 internal protected bool SetMasterClient(int playerId, bool sync)
758 {
759 bool masterReplaced = this.mMasterClient != null && this.mMasterClient.ID != playerId;
760 if (!masterReplaced || !this.mActors.ContainsKey(playerId))
761 {
762 return false;
763 }
764
765 if (sync)
766 {
767 bool sent = this.OpRaiseEvent(PunEvent.AssignMaster, new Hashtable() { { (byte)1, playerId } }, true, null);
768 if (!sent)
769 {
770 return false;
771 }
772 }
773
774 this.hasSwitchedMC = true;
775 this.mMasterClient = this.mActors[playerId];
776 SendMonoMessage(PhotonNetworkingMessage.OnMasterClientSwitched, this.mMasterClient); // we only callback when an actual change is done
777 return true;
778 }
File name: PhotonHandler.cs
Copy
232 internal IEnumerator PingAvailableRegionsCoroutine(bool connectToBest)
233 {
234 BestRegionCodeCurrently = CloudRegionCode.none;
235 while (PhotonNetwork.networkingPeer.AvailableRegions == null)
236 {
237 if (PhotonNetwork.connectionStateDetailed != PeerState.ConnectingToNameServer && PhotonNetwork.connectionStateDetailed != PeerState.ConnectedToNameServer)
238 {
239 Debug.LogError("Call ConnectToNameServer to ping available regions.");
240 yield break; // break if we don't connect to the nameserver at all
241 }
242
243 Debug.Log("Waiting for AvailableRegions. State: " + PhotonNetwork.connectionStateDetailed + " Server: " + PhotonNetwork.Server + " PhotonNetwork.networkingPeer.AvailableRegions " + (PhotonNetwork.networkingPeer.AvailableRegions != null));
244 yield return new WaitForSeconds(0.25f); // wait until pinging finished (offline mode won't ping)
245 }
246
247 if (PhotonNetwork.networkingPeer.AvailableRegions == null || PhotonNetwork.networkingPeer.AvailableRegions.Count == 0)
248 {
249 Debug.LogError("No regions available. Are you sure your appid is valid and setup?");
250 yield break; // break if we don't get regions at all
251 }
252
253 //#if !UNITY_EDITOR && (UNITY_ANDROID || UNITY_IPHONE)
254 //#pragma warning disable 0162 // the library variant defines if we should use PUN's SocketUdp variant (at all)
255 //if (PhotonPeer.NoSocket)
256 //{
257 // if (PhotonNetwork.logLevel >= PhotonLogLevel.Informational)
258 // {
259 // Debug.Log("PUN disconnects to re-use native sockets for pining servers and to find the best.");
260 // }
261 // PhotonNetwork.Disconnect();
262 //}
263 //#pragma warning restore 0162
264 //#endif
265
266 PhotonPingManager pingManager = new PhotonPingManager();
267 foreach (Region region in PhotonNetwork.networkingPeer.AvailableRegions)
268 {
269 SP.StartCoroutine(pingManager.PingSocket(region));
270 }
271
272 while (!pingManager.Done)
273 {
274 yield return new WaitForSeconds(0.1f); // wait until pinging finished (offline mode won't ping)
275 }
276
277
278 Region best = pingManager.BestRegion;
279 PhotonHandler.BestRegionCodeCurrently = best.Code;
280 PhotonHandler.BestRegionCodeInPreferences = best.Code;
281
282 Debug.Log("Found best region: " + best.Code + " ping: " + best.Ping + ". Calling ConnectToRegionMaster() is: " + connectToBest);
283
284
285 if (connectToBest)
286 {
287 PhotonNetwork.networkingPeer.ConnectToRegionMaster(best.Code);
288 }
289 }
File name: PingCloudRegions.cs
Copy
43 public override bool Done()
44 {
45 if (this.GotResult || sock == null)
46 {
47 return true;
48 }
49
50 if (sock.Available <= 0)
51 {
52 return false;
53 }
54
55 int read = sock.Receive(PingBytes, SocketFlags.None);
56 //Debug.Log("Got: " + SupportClass.ByteArrayToString(PingBytes));
57 bool replyMatch = PingBytes[PingBytes.Length - 1] == PingId && read == PingLength;
58 if (!replyMatch) Debug.Log("ReplyMatch is false! ");
59
60
61 this.Successful = read == PingBytes.Length && PingBytes[PingBytes.Length - 1] == PingId;
62 this.GotResult = true;
63 return true;
64 }
Download file with original file name:Done



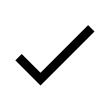
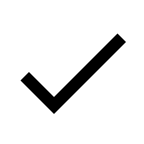
Done 130 lượt xem
Gõ tìm kiếm nhanh...