Format
How do I use Format
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: DemoOwnershipGui.cs
Copy
23 public void OnGUI()
24 {
25 GUI.skin = this.Skin;
26 GUILayout.BeginArea(new Rect(Screen.width - 200, 0, 200, Screen.height));
27 {
28 string label = TransferOwnershipOnRequest ? "passing objects" : "rejecting to pass";
29 if (GUILayout.Button(label))
30 {
31 this.TransferOwnershipOnRequest = !this.TransferOwnershipOnRequest;
32 }
33 }
34 GUILayout.EndArea();
35
36
37
38 if (PhotonNetwork.inRoom)
39 {
40 int playerNr = PhotonNetwork.player.ID;
41 string playerIsMaster = PhotonNetwork.player.isMasterClient ? "(master) " : "";
42 string playerColor = PlayerVariables.GetColorName(PhotonNetwork.player.ID);
43 GUILayout.Label(string.Format("player {0}, {1} {2}(you)", playerNr, playerColor, playerIsMaster));
44
45 foreach (PhotonPlayer otherPlayer in PhotonNetwork.otherPlayers)
46 {
47 playerNr = otherPlayer.ID;
48 playerIsMaster = otherPlayer.isMasterClient ? "(master)" : "";
49 playerColor = PlayerVariables.GetColorName(otherPlayer.ID);
50 GUILayout.Label(string.Format("player {0}, {1} {2}", playerNr, playerColor, playerIsMaster));
51 }
52
53 if (PhotonNetwork.inRoom && PhotonNetwork.otherPlayers.Length == 0)
54 {
55 GUILayout.Label("Join more clients to switch object-control.");
56 }
57 }
58 else
59 {
60 GUILayout.Label(PhotonNetwork.connectionStateDetailed.ToString());
61 }
62 }
File name: ChatGui.cs
Copy
103 public void OnGUI()
104 {
105 if (!this.IsVisible)
106 {
107 return;
108 }
109
110 GUI.skin.label.wordWrap = true;
111 //GUI.skin.button.richText = true; // this allows toolbar buttons to have bold/colored text. nice to indicate new msgs
112 //GUILayout.Button("lala"); // as richText, html tags could be in text
113
114
115 if (Event.current.type == EventType.KeyDown && (Event.current.keyCode == KeyCode.KeypadEnter || Event.current.keyCode == KeyCode.Return))
116 {
117 if ("ChatInput".Equals(GUI.GetNameOfFocusedControl()))
118 {
119 // focus on input -> submit it
120 GuiSendsMsg();
121 return; // showing the now modified list would result in an error. to avoid this, we just skip this single frame
122 }
123 else
124 {
125 // assign focus to input
126 GUI.FocusControl("ChatInput");
127 }
128 }
129
130 GUI.SetNextControlName("");
131 GUILayout.BeginArea(this.GuiRect);
132
133 GUILayout.FlexibleSpace();
134
135 if (this.chatClient.State != ChatState.ConnectedToFrontEnd)
136 {
137 GUILayout.Label("Not in chat yet.");
138 }
139 else
140 {
141 List
142 int countOfPublicChannels = channels.Count;
143 channels.AddRange(this.chatClient.PrivateChannels.Keys);
144
145 if (channels.Count > 0)
146 {
147 int previouslySelectedChannelIndex = this.selectedChannelIndex;
148 int channelIndex = channels.IndexOf(this.selectedChannelName);
149 this.selectedChannelIndex = (channelIndex >= 0) ? channelIndex : 0;
150
151 this.selectedChannelIndex = GUILayout.Toolbar(this.selectedChannelIndex, channels.ToArray(), GUILayout.ExpandWidth(false));
152 this.scrollPos = GUILayout.BeginScrollView(this.scrollPos);
153
154 this.doingPrivateChat = (this.selectedChannelIndex >= countOfPublicChannels);
155 this.selectedChannelName = channels[this.selectedChannelIndex];
156
157 if (this.selectedChannelIndex != previouslySelectedChannelIndex)
158 {
159 // changed channel -> scroll down, if private: pre-fill "to" field with target user's name
160 this.scrollPos.y = float.MaxValue;
161 if (this.doingPrivateChat)
162 {
163 string[] pieces = this.selectedChannelName.Split(new char[] {':'}, 3);
164 this.userIdInput = pieces[1];
165 }
166 }
167
168 GUILayout.Label(ChatGui.WelcomeText);
169
170 if (this.chatClient.TryGetChannel(selectedChannelName, this.doingPrivateChat, out this.selectedChannel))
171 {
172 for (int i = 0; i < this.selectedChannel.Messages.Count; i++)
173 {
174 string sender = this.selectedChannel.Senders[i];
175 object message = this.selectedChannel.Messages[i];
176 GUILayout.Label(string.Format("{0}: {1}", sender, message));
177 }
178 }
179
180 GUILayout.EndScrollView();
181 }
182 }
183
184
185 GUILayout.BeginHorizontal();
186 if (doingPrivateChat)
187 {
188 GUILayout.Label("to:", GUILayout.ExpandWidth(false));
189 GUI.SetNextControlName("WhisperTo");
190 this.userIdInput = GUILayout.TextField(this.userIdInput, GUILayout.MinWidth(100), GUILayout.ExpandWidth(false));
191 string focussed = GUI.GetNameOfFocusedControl();
192 if (focussed.Equals("WhisperTo"))
193 {
194 if (this.userIdInput.Equals("username"))
195 {
196 this.userIdInput = "";
197 }
198 }
199 else if (string.IsNullOrEmpty(this.userIdInput))
200 {
201 this.userIdInput = "username";
202 }
203
204 }
205 GUI.SetNextControlName("ChatInput");
206 inputLine = GUILayout.TextField(inputLine);
207 if (GUILayout.Button("Send", GUILayout.ExpandWidth(false)))
208 {
209 GuiSendsMsg();
210 }
211 GUILayout.EndHorizontal();
212 GUILayout.EndArea();
213 }
File name: ChatGui.cs
Copy
348 public void OnStatusUpdate(string user, int status, bool gotMessage, object message)
349 {
350 // this is how you get status updates of friends.
351 // this demo simply adds status updates to the currently shown chat.
352 // you could buffer them or use them any other way, too.
353
354 ChatChannel activeChannel = this.selectedChannel;
355 if (activeChannel != null)
356 {
357 activeChannel.Add("info", string.Format("{0} is {1}. Msg:{2}", user, status, message));
358 }
359
360 Debug.LogWarning("status: " + string.Format("{0} is {1}. Msg:{2}", user, status, message));
361 }
File name: WorkerMenu.cs
Copy
65 public void OnGUI()
66 {
67 if (this.Skin != null)
68 {
69 GUI.skin = this.Skin;
70 }
71
72 if (!PhotonNetwork.connected)
73 {
74 if (PhotonNetwork.connecting)
75 {
76 GUILayout.Label("Connecting to: " + PhotonNetwork.ServerAddress);
77 }
78 else
79 {
80 GUILayout.Label("Not connected. Check console output. Detailed connection state: " + PhotonNetwork.connectionStateDetailed + " Server: " + PhotonNetwork.ServerAddress);
81 }
82
83 if (this.connectFailed)
84 {
85 GUILayout.Label("Connection failed. Check setup and use Setup Wizard to fix configuration.");
86 GUILayout.Label(String.Format("Server: {0}", new object[] {PhotonNetwork.ServerAddress}));
87 GUILayout.Label("AppId: " + PhotonNetwork.PhotonServerSettings.AppID);
88
89 if (GUILayout.Button("Try Again", GUILayout.Width(100)))
90 {
91 this.connectFailed = false;
92 PhotonNetwork.ConnectUsingSettings("0.9");
93 }
94 }
95
96 return;
97 }
98
99 Rect content = new Rect((Screen.width - WidthAndHeight.x)/2, (Screen.height - WidthAndHeight.y)/2, WidthAndHeight.x, WidthAndHeight.y);
100 GUI.Box(content,"Join or Create Room");
101 GUILayout.BeginArea(content);
102
103 GUILayout.Space(40);
104
105 // Player name
106 GUILayout.BeginHorizontal();
107 GUILayout.Label("Player name:", GUILayout.Width(150));
108 PhotonNetwork.playerName = GUILayout.TextField(PhotonNetwork.playerName);
109 GUILayout.Space(158);
110 if (GUI.changed)
111 {
112 // Save name
113 PlayerPrefs.SetString("playerName", PhotonNetwork.playerName);
114 }
115 GUILayout.EndHorizontal();
116
117 GUILayout.Space(15);
118
119 // Join room by title
120 GUILayout.BeginHorizontal();
121 GUILayout.Label("Roomname:", GUILayout.Width(150));
122 this.roomName = GUILayout.TextField(this.roomName);
123
124 if (GUILayout.Button("Create Room", GUILayout.Width(150)))
125 {
126 PhotonNetwork.CreateRoom(this.roomName, new RoomOptions() { maxPlayers = 10 }, null);
127 }
128
129 GUILayout.EndHorizontal();
130
131 // Create a room (fails if exist!)
132 GUILayout.BeginHorizontal();
133 GUILayout.FlexibleSpace();
134 //this.roomName = GUILayout.TextField(this.roomName);
135 if (GUILayout.Button("Join Room", GUILayout.Width(150)))
136 {
137 PhotonNetwork.JoinRoom(this.roomName);
138 }
139
140 GUILayout.EndHorizontal();
141
142
143 if (!string.IsNullOrEmpty(this.ErrorDialog))
144 {
145 GUILayout.Label(this.ErrorDialog);
146
147 if (timeToClearDialog < Time.time)
148 {
149 timeToClearDialog = 0;
150 this.ErrorDialog = "";
151 }
152 }
153
154 GUILayout.Space(15);
155
156 // Join random room
157 GUILayout.BeginHorizontal();
158
159 GUILayout.Label(PhotonNetwork.countOfPlayers + " users are online in " + PhotonNetwork.countOfRooms + " rooms.");
160 GUILayout.FlexibleSpace();
161 if (GUILayout.Button("Join Random", GUILayout.Width(150)))
162 {
163 PhotonNetwork.JoinRandomRoom();
164 }
165
166
167 GUILayout.EndHorizontal();
168
169 GUILayout.Space(15);
170 if (PhotonNetwork.GetRoomList().Length == 0)
171 {
172 GUILayout.Label("Currently no games are available.");
173 GUILayout.Label("Rooms will be listed here, when they become available.");
174 }
175 else
176 {
177 GUILayout.Label(PhotonNetwork.GetRoomList().Length + " rooms available:");
178
179 // Room listing: simply call GetRoomList: no need to fetch/poll whatever!
180 this.scrollPos = GUILayout.BeginScrollView(this.scrollPos);
181 foreach (RoomInfo roomInfo in PhotonNetwork.GetRoomList())
182 {
183 GUILayout.BeginHorizontal();
184 GUILayout.Label(roomInfo.name + " " + roomInfo.playerCount + "/" + roomInfo.maxPlayers);
185 if (GUILayout.Button("Join", GUILayout.Width(150)))
186 {
187 PhotonNetwork.JoinRoom(roomInfo.name);
188 }
189
190 GUILayout.EndHorizontal();
191 }
192
193 GUILayout.EndScrollView();
194 }
195
196 GUILayout.EndArea();
197 }
File name: AccountService.cs
Copy
161 private Uri RegistrationUri(string email, byte origin)
162 {
163 string emailEncoded = Uri.EscapeDataString(email);
164 string uriString = string.Format("{0}?email={1}&origin={2}", ServiceUrl, emailEncoded, origin);
165
166 return new Uri(uriString);
167 }
File name: ReorderableListResources.cs
Copy
147 public static Texture2D CreatePixelTexture( string name, Color color )
148 {
149 var tex = new Texture2D( 1, 1, TextureFormat.ARGB32, false, true );
150 tex.name = name;
151 tex.hideFlags = HideFlags.HideAndDontSave;
152 tex.filterMode = FilterMode.Point;
153 tex.SetPixel( 0, 0, color );
154 tex.Apply();
155 return tex;
156 }
File name: ReorderableListResources.cs
Copy
168 private static void LoadResourceAssets()
169 {
170 var skin = EditorGUIUtility.isProSkin ? s_DarkSkin : s_LightSkin;
171 s_Cached = new Texture2D[ skin.Length ];
172
173 for( int i = 0; i < s_Cached.Length; ++i )
174 {
175 // Get image data (PNG) from base64 encoded strings.
176 byte[] imageData = Convert.FromBase64String( skin[ i ] );
177
178 // Gather image size from image data.
179 int texWidth, texHeight;
180 GetImageSize( imageData, out texWidth, out texHeight );
181
182 // Generate texture asset.
183 var tex = new Texture2D( texWidth, texHeight, TextureFormat.ARGB32, false, true );
184 tex.hideFlags = HideFlags.HideAndDontSave;
185 tex.name = "(Generated) ReorderableList:" + i;
186 tex.filterMode = FilterMode.Point;
187 tex.LoadImage( imageData );
188
189 s_Cached[ i ] = tex;
190 }
191
192 s_LightSkin = null;
193 s_DarkSkin = null;
194 }
File name: FriendInfo.cs
Copy
12 public override string ToString()
13 {
14 return string.Format("{0}\t is: {1}", this.Name, (!this.IsOnline) ? "offline" : this.IsInRoom ? "playing" : "on master");
15 }
File name: LoadbalancingPeer.cs
Copy
86 public virtual bool OpCreateRoom(string roomName, RoomOptions roomOptions, TypedLobby lobby, Hashtable playerProperties, bool onGameServer)
87 {
88 if (this.DebugOut >= DebugLevel.INFO)
89 {
90 this.Listener.DebugReturn(DebugLevel.INFO, "OpCreateRoom()");
91 }
92
93 Dictionary
94
95 if (!string.IsNullOrEmpty(roomName))
96 {
97 op[ParameterCode.RoomName] = roomName;
98 }
99 if (lobby != null)
100 {
101 op[ParameterCode.LobbyName] = lobby.Name;
102 op[ParameterCode.LobbyType] = (byte)lobby.Type;
103 }
104
105 if (onGameServer)
106 {
107 if (playerProperties != null && playerProperties.Count > 0)
108 {
109 op[ParameterCode.PlayerProperties] = playerProperties;
110 op[ParameterCode.Broadcast] = true; // TODO: check if this also makes sense when creating a room?! // broadcast actor properties
111 }
112
113
114 if (roomOptions == null)
115 {
116 roomOptions = new RoomOptions();
117 }
118
119 Hashtable gameProperties = new Hashtable();
120 op[ParameterCode.GameProperties] = gameProperties;
121 gameProperties.MergeStringKeys(roomOptions.customRoomProperties);
122
123 gameProperties[GameProperties.IsOpen] = roomOptions.isOpen; // TODO: check default value. dont send this then
124 gameProperties[GameProperties.IsVisible] = roomOptions.isVisible; // TODO: check default value. dont send this then
125 gameProperties[GameProperties.PropsListedInLobby] = roomOptions.customRoomPropertiesForLobby;
126 if (roomOptions.maxPlayers > 0)
127 {
128 gameProperties[GameProperties.MaxPlayers] = roomOptions.maxPlayers;
129 }
130 if (roomOptions.cleanupCacheOnLeave)
131 {
132 op[ParameterCode.CleanupCacheOnLeave] = true; // this is actually setting the room's config
133 gameProperties[GameProperties.CleanupCacheOnLeave] = true; // this is only informational for the clients which join
134 }
135 }
136
137 // UnityEngine.Debug.Log("CreateGame: " + SupportClass.DictionaryToString(op));
138 return this.OpCustom(OperationCode.CreateGame, op, true);
139 }
File name: LoadbalancingPeer.cs
Copy
143 public virtual bool OpJoinRoom(string roomName, RoomOptions roomOptions, TypedLobby lobby, bool createIfNotExists, Hashtable playerProperties, bool onGameServer)
144 {
145 Dictionary
146
147 if (!string.IsNullOrEmpty(roomName))
148 {
149 op[ParameterCode.RoomName] = roomName;
150 }
151 if (createIfNotExists)
152 {
153 op[ParameterCode.CreateIfNotExists] = true;
154 if (lobby != null)
155 {
156 op[ParameterCode.LobbyName] = lobby.Name;
157 op[ParameterCode.LobbyType] = (byte)lobby.Type;
158 }
159 }
160
161 if (onGameServer)
162 {
163 if (playerProperties != null && playerProperties.Count > 0)
164 {
165 op[ParameterCode.PlayerProperties] = playerProperties;
166 op[ParameterCode.Broadcast] = true; // broadcast actor properties
167 }
168
169
170 if (createIfNotExists)
171 {
172 if (roomOptions == null)
173 {
174 roomOptions = new RoomOptions();
175 }
176
177 Hashtable gameProperties = new Hashtable();
178 op[ParameterCode.GameProperties] = gameProperties;
179 gameProperties.MergeStringKeys(roomOptions.customRoomProperties);
180
181 gameProperties[GameProperties.IsOpen] = roomOptions.isOpen;
182 gameProperties[GameProperties.IsVisible] = roomOptions.isVisible;
183 gameProperties[GameProperties.PropsListedInLobby] = roomOptions.customRoomPropertiesForLobby;
184 if (roomOptions.maxPlayers > 0)
185 {
186 gameProperties[GameProperties.MaxPlayers] = roomOptions.maxPlayers;
187 }
188 if (roomOptions.cleanupCacheOnLeave)
189 {
190 op[ParameterCode.CleanupCacheOnLeave] = true; // this is actually setting the room's config
191 gameProperties[GameProperties.CleanupCacheOnLeave] = true; // this is only informational for the clients which join
192 }
193 }
194 }
195
196 // UnityEngine.Debug.Log("JoinGame: " + SupportClass.DictionaryToString(op));
197 return this.OpCustom(OperationCode.JoinGame, op, true);
198 }
Download file with original file name:Format
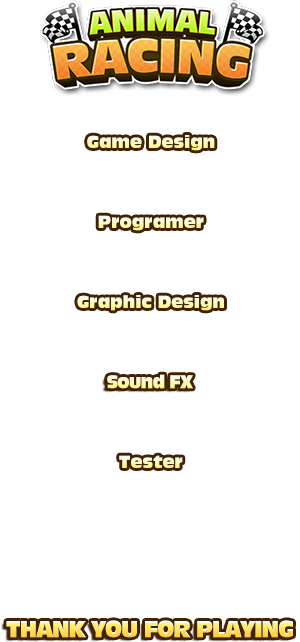
Format 105 lượt xem
Gõ tìm kiếm nhanh...