Point
How do I use Point
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: PickupCamera.cs
Copy
198 void SetUpRotation( Vector3 centerPos, Vector3 headPos )
199 {
200 // Now it's getting hairy. The devil is in the details here, the big issue is jumping of course.
201 // * When jumping up and down we don't want to center the guy in screen space.
202 // This is important to give a feel for how high you jump and avoiding large camera movements.
203 //
204 // * At the same time we dont want him to ever go out of screen and we want all rotations to be totally smooth.
205 //
206 // So here is what we will do:
207 //
208 // 1. We first find the rotation around the y axis. Thus he is always centered on the y-axis
209 // 2. When grounded we make him be centered
210 // 3. When jumping we keep the camera rotation but rotate the camera to get him back into view if his head is above some threshold
211 // 4. When landing we smoothly interpolate towards centering him on screen
212 Vector3 cameraPos = cameraTransform.position;
213 Vector3 offsetToCenter = centerPos - cameraPos;
214
215 // Generate base rotation only around y-axis
216 Quaternion yRotation = Quaternion.LookRotation( new Vector3( offsetToCenter.x, 0, offsetToCenter.z ) );
217
218 Vector3 relativeOffset = Vector3.forward * distance + Vector3.down * height;
219 cameraTransform.rotation = yRotation * Quaternion.LookRotation( relativeOffset );
220
221 // Calculate the projected center position and top position in world space
222 Ray centerRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, 0.5f, 1 ) );
223 Ray topRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, clampHeadPositionScreenSpace, 1 ) );
224
225 Vector3 centerRayPos = centerRay.GetPoint( distance );
226 Vector3 topRayPos = topRay.GetPoint( distance );
227
228 float centerToTopAngle = Vector3.Angle( centerRay.direction, topRay.direction );
229
230 float heightToAngle = centerToTopAngle / ( centerRayPos.y - topRayPos.y );
231
232 float extraLookAngle = heightToAngle * ( centerRayPos.y - centerPos.y );
233 if( extraLookAngle < centerToTopAngle )
234 {
235 extraLookAngle = 0;
236 }
237 else
238 {
239 extraLookAngle = extraLookAngle - centerToTopAngle;
240 cameraTransform.rotation *= Quaternion.Euler( -extraLookAngle, 0, 0 );
241 }
242 }
File name: RPGCamera.cs
Copy
20 void Start()
21 {
22 m_CameraTransform = transform.GetChild( 0 );
23 m_LocalForwardVector = m_CameraTransform.forward;
24
25 m_Distance = -m_CameraTransform.localPosition.z / m_CameraTransform.forward.z;
26 m_Distance = Mathf.Clamp( m_Distance, MinimumDistance, MaximumDistance );
27 m_LookAtPoint = m_CameraTransform.localPosition + m_LocalForwardVector * m_Distance;
28 }
File name: RPGCamera.cs
Copy
43 void UpdateZoom()
44 {
45 m_CameraTransform.localPosition = m_LookAtPoint - m_LocalForwardVector * m_Distance;
46 }
File name: ThirdPersonCamera.cs
Copy
190 void SetUpRotation( Vector3 centerPos, Vector3 headPos )
191 {
192 // Now it's getting hairy. The devil is in the details here, the big issue is jumping of course.
193 // * When jumping up and down we don't want to center the guy in screen space.
194 // This is important to give a feel for how high you jump and avoiding large camera movements.
195 //
196 // * At the same time we dont want him to ever go out of screen and we want all rotations to be totally smooth.
197 //
198 // So here is what we will do:
199 //
200 // 1. We first find the rotation around the y axis. Thus he is always centered on the y-axis
201 // 2. When grounded we make him be centered
202 // 3. When jumping we keep the camera rotation but rotate the camera to get him back into view if his head is above some threshold
203 // 4. When landing we smoothly interpolate towards centering him on screen
204 Vector3 cameraPos = cameraTransform.position;
205 Vector3 offsetToCenter = centerPos - cameraPos;
206
207 // Generate base rotation only around y-axis
208 Quaternion yRotation = Quaternion.LookRotation( new Vector3( offsetToCenter.x, 0, offsetToCenter.z ) );
209
210 Vector3 relativeOffset = Vector3.forward * distance + Vector3.down * height;
211 cameraTransform.rotation = yRotation * Quaternion.LookRotation( relativeOffset );
212
213 // Calculate the projected center position and top position in world space
214 Ray centerRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, 0.5f, 1 ) );
215 Ray topRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, clampHeadPositionScreenSpace, 1 ) );
216
217 Vector3 centerRayPos = centerRay.GetPoint( distance );
218 Vector3 topRayPos = topRay.GetPoint( distance );
219
220 float centerToTopAngle = Vector3.Angle( centerRay.direction, topRay.direction );
221
222 float heightToAngle = centerToTopAngle / ( centerRayPos.y - topRayPos.y );
223
224 float extraLookAngle = heightToAngle * ( centerRayPos.y - centerPos.y );
225 if( extraLookAngle < centerToTopAngle )
226 {
227 extraLookAngle = 0;
228 }
229 else
230 {
231 extraLookAngle = extraLookAngle - centerToTopAngle;
232 cameraTransform.rotation *= Quaternion.Euler( -extraLookAngle, 0, 0 );
233 }
234 }
File name: ClickDetector.cs
Copy
7 void Update()
8 {
9 // if this player is not "it", the player can't tag anyone, so don't do anything on collision
10 if (PhotonNetwork.player.ID != GameLogic.playerWhoIsIt)
11 {
12 return;
13 }
14
15 if (Input.GetButton("Fire1"))
16 {
17 GameObject goPointedAt = RaycastObject(Input.mousePosition);
18
19 if (goPointedAt != null && goPointedAt != this.gameObject && goPointedAt.name.Equals("monsterprefab(Clone)", StringComparison.OrdinalIgnoreCase))
20 {
21 PhotonView rootView = goPointedAt.transform.root.GetComponent
22 GameLogic.TagPlayer(rootView.owner.ID);
23 }
24 }
25 }
File name: ClickDetector.cs
Copy
27 private GameObject RaycastObject(Vector2 screenPos)
28 {
29 RaycastHit info;
30 #if UNITY_3_5
31 Camera cam = Camera.mainCamera;
32 #else
33 Camera cam = Camera.main;
34 #endif
35
36 if (Physics.Raycast(cam.ScreenPointToRay(screenPos), out info, 200))
37 {
38 return info.collider.gameObject;
39 }
40
41 return null;
42 }
File name: AccountService.cs
Copy
42 public AccountService()
43 {
44 WebRequest.DefaultWebProxy = null;
45 ServicePointManager.ServerCertificateValidationCallback = Validator;
46 }
File name: ReorderableListResources.cs
Copy
147 public static Texture2D CreatePixelTexture( string name, Color color )
148 {
149 var tex = new Texture2D( 1, 1, TextureFormat.ARGB32, false, true );
150 tex.name = name;
151 tex.hideFlags = HideFlags.HideAndDontSave;
152 tex.filterMode = FilterMode.Point;
153 tex.SetPixel( 0, 0, color );
154 tex.Apply();
155 return tex;
156 }
File name: ReorderableListResources.cs
Copy
168 private static void LoadResourceAssets()
169 {
170 var skin = EditorGUIUtility.isProSkin ? s_DarkSkin : s_LightSkin;
171 s_Cached = new Texture2D[ skin.Length ];
172
173 for( int i = 0; i < s_Cached.Length; ++i )
174 {
175 // Get image data (PNG) from base64 encoded strings.
176 byte[] imageData = Convert.FromBase64String( skin[ i ] );
177
178 // Gather image size from image data.
179 int texWidth, texHeight;
180 GetImageSize( imageData, out texWidth, out texHeight );
181
182 // Generate texture asset.
183 var tex = new Texture2D( texWidth, texHeight, TextureFormat.ARGB32, false, true );
184 tex.hideFlags = HideFlags.HideAndDontSave;
185 tex.name = "(Generated) ReorderableList:" + i;
186 tex.filterMode = FilterMode.Point;
187 tex.LoadImage( imageData );
188
189 s_Cached[ i ] = tex;
190 }
191
192 s_LightSkin = null;
193 s_DarkSkin = null;
194 }
File name: HighlightOwnedGameObj.cs
Copy
12 private void Update()
13 {
14 if (photonView.isMine)
15 {
16 if (this.markerTransform == null)
17 {
18 GameObject markerObject = (GameObject) GameObject.Instantiate(this.PointerPrefab);
19 markerObject.transform.parent = gameObject.transform;
20 this.markerTransform = markerObject.transform;
21 }
22
23 Vector3 parentPos = gameObject.transform.position;
24 this.markerTransform.position = new Vector3(parentPos.x, parentPos.y + this.Offset, parentPos.z);
25 this.markerTransform.rotation = Quaternion.identity;
26 }
27 else if (this.markerTransform != null)
28 {
29 Destroy(this.markerTransform.gameObject);
30 this.markerTransform = null;
31 }
32 }
Download file with original file name:Point
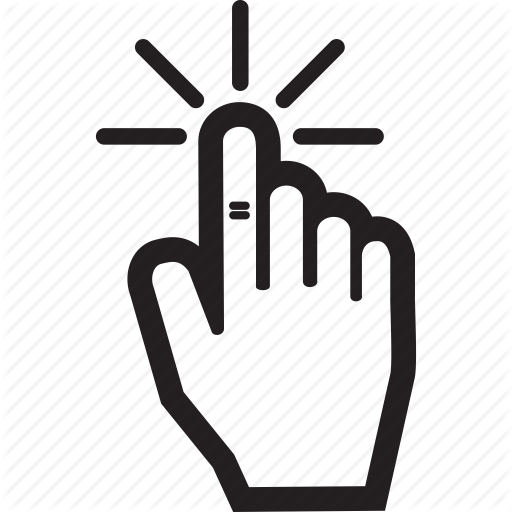
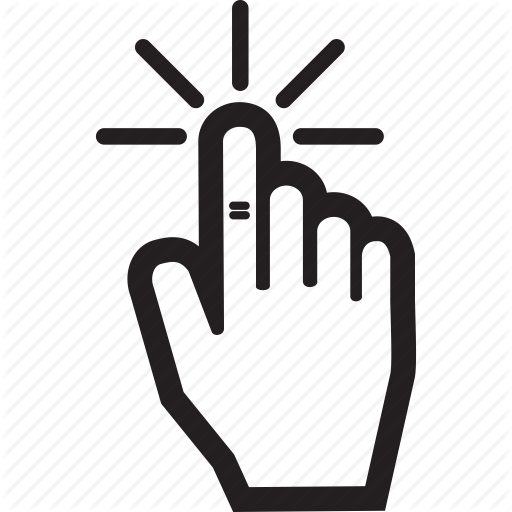
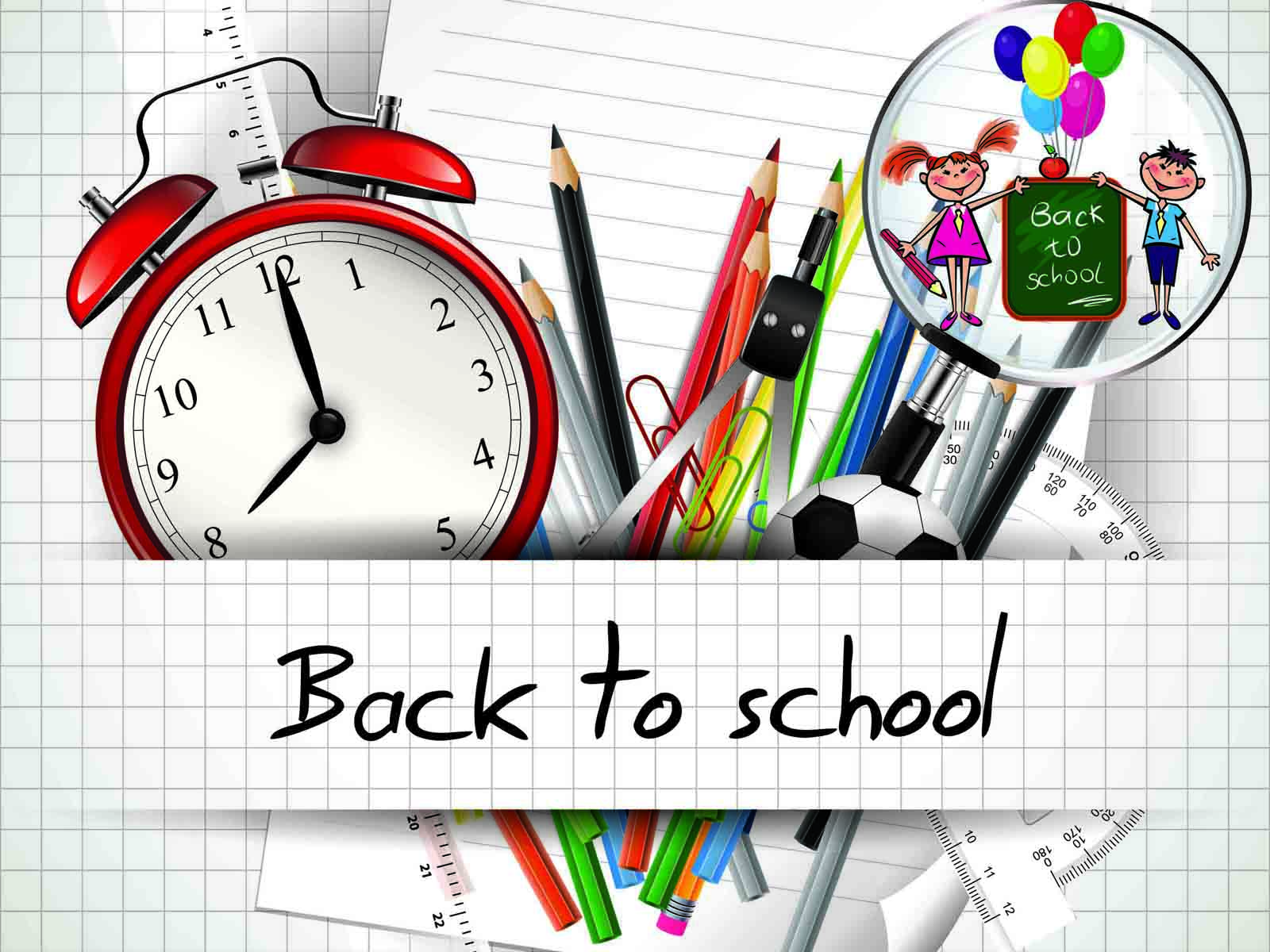
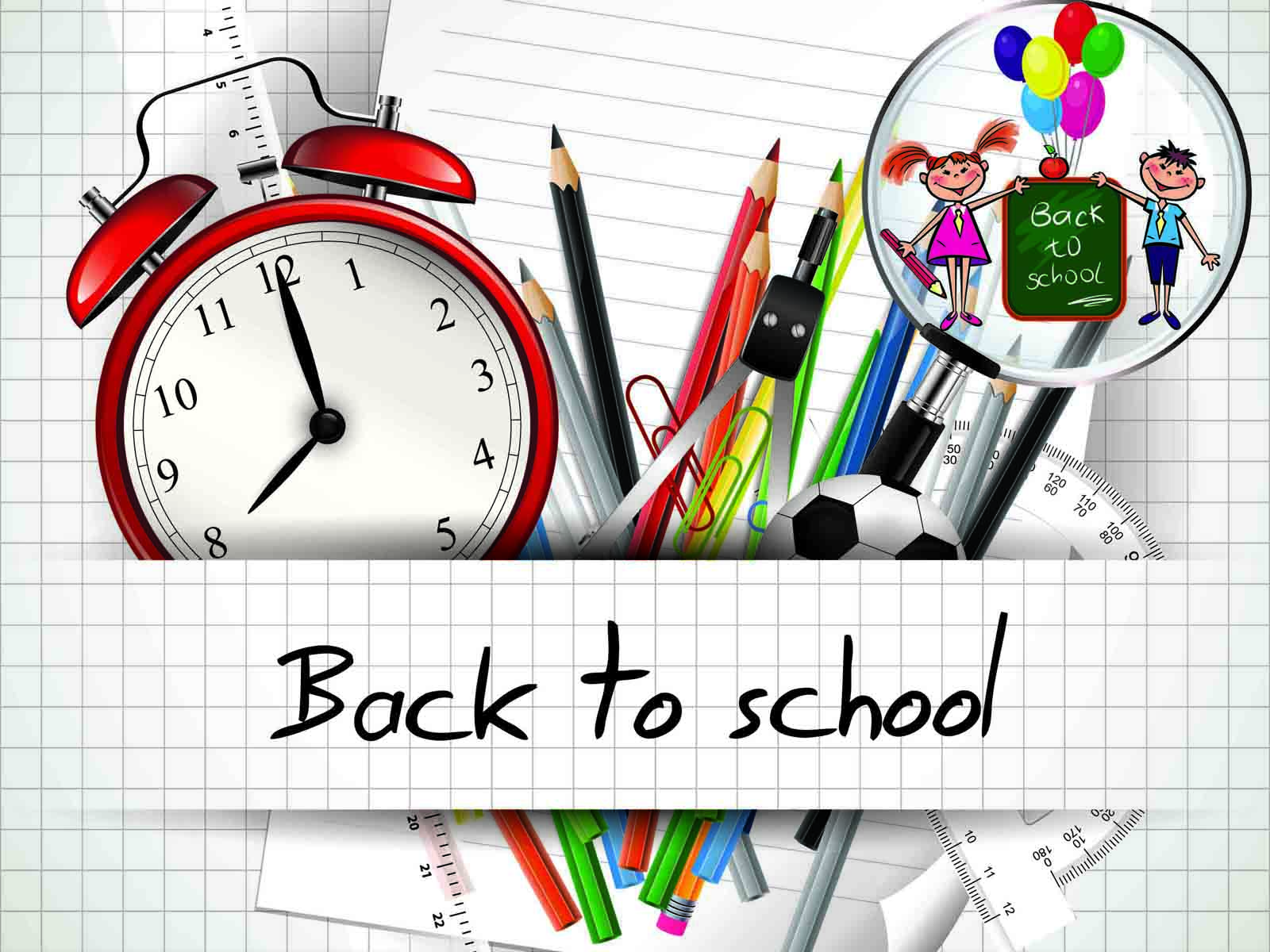
Point 117 lượt xem
Gõ tìm kiếm nhanh...