Round
How do I use Round
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: JumpAndRunMovement.cs
Copy
22 void Update()
23 {
24 UpdateIsGrounded();
25 UpdateIsRunning();
26 UpdateFacingDirection();
27 }
File name: JumpAndRunMovement.cs
Copy
52 void UpdateJumping()
53 {
54 if( Input.GetKey( KeyCode.Space ) == true && m_IsGrounded == true )
55 {
56 m_Animator.SetTrigger( "IsJumping" );
57 m_Body.AddForce( Vector2.up * JumpForce );
58 m_PhotonView.RPC( "DoJump", PhotonTargets.Others );
59 }
60 }
File name: JumpAndRunMovement.cs
Copy
94 void UpdateIsGrounded()
95 {
96 Vector2 position = new Vector2( transform.position.x, transform.position.y );
97
98 RaycastHit2D hit = Physics2D.Raycast( position, -Vector2.up, 0.1f, 1 << LayerMask.NameToLayer( "Ground" ) );
99
100 m_IsGrounded = hit.collider != null;
101 m_Animator.SetBool( "IsGrounded", m_IsGrounded );
102 }
File name: ChatGui.cs
Copy
58 public void Start()
59 {
60 DontDestroyOnLoad(this.gameObject);
61 Application.runInBackground = true; // this must run in background or it will drop connection if not focussed.
62
63 if (string.IsNullOrEmpty(this.UserName))
64 {
65 this.UserName = "user" + Environment.TickCount%99; //made-up username
66 }
67
68 chatClient = new ChatClient(this);
69 chatClient.Connect(ChatAppId, "1.0", this.UserName, null);
70
71 if (this.AlignBottom)
72 {
73 this.GuiRect.y = Screen.height - this.GuiRect.height;
74 }
75 if (this.FullScreen)
76 {
77 this.GuiRect.x = 0;
78 this.GuiRect.y = 0;
79 this.GuiRect.width = Screen.width;
80 this.GuiRect.height = Screen.height;
81 }
82
83 Debug.Log(this.UserName);
84 }
File name: PickupController.cs
Copy
151 void Update()
152 {
153 if (isControllable)
154 {
155 if (Input.GetButtonDown("Jump"))
156 {
157 lastJumpButtonTime = Time.time;
158 }
159
160 UpdateSmoothedMovementDirection();
161
162 // Apply gravity
163 // - extra power jump modifies gravity
164 // - controlledDescent mode modifies gravity
165 ApplyGravity();
166
167 // Apply jumping logic
168 ApplyJumping();
169
170
171 // Calculate actual motion
172 Vector3 movement = moveDirection * moveSpeed + new Vector3(0, verticalSpeed, 0) + inAirVelocity;
173 movement *= Time.deltaTime;
174
175 //Debug.Log(movement.x.ToString("0.000") + ":" + movement.z.ToString("0.000"));
176
177 // Move the controller
178 CharacterController controller = GetComponent
179 collisionFlags = controller.Move(movement);
180
181 }
182
183 // PUN: if a remote position is known, we smooth-move to it (being late(r) but smoother)
184 if (this.remotePosition != Vector3.zero)
185 {
186 transform.position = Vector3.Lerp(transform.position, this.remotePosition, Time.deltaTime * this.RemoteSmoothing);
187 }
188
189 velocity = (transform.position - lastPos)*25;
190
191 // ANIMATION sector
192 if (_animation)
193 {
194 if (_characterState == PickupCharacterState.Jumping)
195 {
196 if (!jumpingReachedApex)
197 {
198 _animation[jumpPoseAnimation.name].speed = jumpAnimationSpeed;
199 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
200 _animation.CrossFade(jumpPoseAnimation.name);
201 }
202 else
203 {
204 _animation[jumpPoseAnimation.name].speed = -landAnimationSpeed;
205 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
206 _animation.CrossFade(jumpPoseAnimation.name);
207 }
208 }
209 else
210 {
211 if (_characterState == PickupCharacterState.Idle)
212 {
213 _animation.CrossFade(idleAnimation.name);
214 }
215 else if (_characterState == PickupCharacterState.Running)
216 {
217 _animation[runAnimation.name].speed = runMaxAnimationSpeed;
218 if (this.isControllable)
219 {
220 _animation[runAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, runMaxAnimationSpeed);
221 }
222 _animation.CrossFade(runAnimation.name);
223 }
224 else if (_characterState == PickupCharacterState.Trotting)
225 {
226 _animation[walkAnimation.name].speed = trotMaxAnimationSpeed;
227 if (this.isControllable)
228 {
229 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, trotMaxAnimationSpeed);
230 }
231 _animation.CrossFade(walkAnimation.name);
232 }
233 else if (_characterState == PickupCharacterState.Walking)
234 {
235 _animation[walkAnimation.name].speed = walkMaxAnimationSpeed;
236 if (this.isControllable)
237 {
238 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, walkMaxAnimationSpeed);
239 }
240 _animation.CrossFade(walkAnimation.name);
241 }
242
243 if (_characterState != PickupCharacterState.Running)
244 {
245 _animation[runAnimation.name].time = 0.0f;
246 }
247 }
248 }
249 // ANIMATION sector
250
251 // Set rotation to the move direction
252 if (IsGrounded())
253 {
254 // a specialty of this controller: you can disable rotation!
255 if (DoRotate)
256 {
257 transform.rotation = Quaternion.LookRotation(moveDirection);
258 }
259 }
260 else
261 {
262 /* This causes choppy behaviour when colliding with SIDES
263 * Vector3 xzMove = velocity;
264 xzMove.y = 0;
265 if (xzMove.sqrMagnitude > 0.001f)
266 {
267 transform.rotation = Quaternion.LookRotation(xzMove);
268 }*/
269 }
270
271 // We are in jump mode but just became grounded
272 if (IsGrounded())
273 {
274 lastGroundedTime = Time.time;
275 inAirVelocity = Vector3.zero;
276 if (jumping)
277 {
278 jumping = false;
279 SendMessage("DidLand", SendMessageOptions.DontRequireReceiver);
280 }
281 }
282
283 lastPos = transform.position;
284 }
File name: PickupController.cs
Copy
308 void UpdateSmoothedMovementDirection()
309 {
310 Transform cameraTransform = Camera.main.transform;
311 bool grounded = IsGrounded();
312
313 // Forward vector relative to the camera along the x-z plane
314 Vector3 forward = cameraTransform.TransformDirection(Vector3.forward);
315 forward.y = 0;
316 forward = forward.normalized;
317
318 // Right vector relative to the camera
319 // Always orthogonal to the forward vector
320 Vector3 right = new Vector3(forward.z, 0, -forward.x);
321
322 float v = Input.GetAxisRaw("Vertical");
323 float h = Input.GetAxisRaw("Horizontal");
324
325 // Are we moving backwards or looking backwards
326 if (v < -0.2f)
327 movingBack = true;
328 else
329 movingBack = false;
330
331 bool wasMoving = isMoving;
332 isMoving = Mathf.Abs(h) > 0.1f || Mathf.Abs(v) > 0.1f;
333
334 // Target direction relative to the camera
335 Vector3 targetDirection = h * right + v * forward;
336 // Debug.Log("targetDirection " + targetDirection);
337
338 // Grounded controls
339 if (grounded)
340 {
341 // Lock camera for short period when transitioning moving & standing still
342 lockCameraTimer += Time.deltaTime;
343 if (isMoving != wasMoving)
344 lockCameraTimer = 0.0f;
345
346 // We store speed and direction seperately,
347 // so that when the character stands still we still have a valid forward direction
348 // moveDirection is always normalized, and we only update it if there is user input.
349 if (targetDirection != Vector3.zero)
350 {
351 // If we are really slow, just snap to the target direction
352 if (moveSpeed < walkSpeed * 0.9f && grounded)
353 {
354 moveDirection = targetDirection.normalized;
355 }
356 // Otherwise smoothly turn towards it
357 else
358 {
359 moveDirection = Vector3.RotateTowards(moveDirection, targetDirection, rotateSpeed * Mathf.Deg2Rad * Time.deltaTime, 1000);
360
361 moveDirection = moveDirection.normalized;
362 }
363 }
364
365 // Smooth the speed based on the current target direction
366 float curSmooth = speedSmoothing * Time.deltaTime;
367
368 // Choose target speed
369 //* We want to support analog input but make sure you cant walk faster diagonally than just forward or sideways
370 float targetSpeed = Mathf.Min(targetDirection.magnitude, 1.0f);
371
372 _characterState = PickupCharacterState.Idle;
373
374 // Pick speed modifier
375 if ((Input.GetKey(KeyCode.LeftShift) | Input.GetKey(KeyCode.RightShift)) && isMoving)
376 {
377 targetSpeed *= runSpeed;
378 _characterState = PickupCharacterState.Running;
379 }
380 else if (Time.time - trotAfterSeconds > walkTimeStart)
381 {
382 targetSpeed *= trotSpeed;
383 _characterState = PickupCharacterState.Trotting;
384 }
385 else if (isMoving)
386 {
387 targetSpeed *= walkSpeed;
388 _characterState = PickupCharacterState.Walking;
389 }
390
391 moveSpeed = Mathf.Lerp(moveSpeed, targetSpeed, curSmooth);
392
393 // Reset walk time start when we slow down
394 if (moveSpeed < walkSpeed * 0.3f)
395 walkTimeStart = Time.time;
396 }
397 // In air controls
398 else
399 {
400 // Lock camera while in air
401 if (jumping)
402 lockCameraTimer = 0.0f;
403
404 if (isMoving)
405 inAirVelocity += targetDirection.normalized * Time.deltaTime * inAirControlAcceleration;
406 }
407 }
File name: ThirdPersonController.cs
Copy
131 void UpdateSmoothedMovementDirection()
132 {
133 Transform cameraTransform = Camera.main.transform;
134 bool grounded = IsGrounded();
135
136 // Forward vector relative to the camera along the x-z plane
137 Vector3 forward = cameraTransform.TransformDirection(Vector3.forward);
138 forward.y = 0;
139 forward = forward.normalized;
140
141 // Right vector relative to the camera
142 // Always orthogonal to the forward vector
143 Vector3 right = new Vector3(forward.z, 0, -forward.x);
144
145 float v = Input.GetAxisRaw("Vertical");
146 float h = Input.GetAxisRaw("Horizontal");
147
148 // Are we moving backwards or looking backwards
149 if (v < -0.2f)
150 movingBack = true;
151 else
152 movingBack = false;
153
154 bool wasMoving = isMoving;
155 isMoving = Mathf.Abs(h) > 0.1f || Mathf.Abs(v) > 0.1f;
156
157 // Target direction relative to the camera
158 Vector3 targetDirection = h * right + v * forward;
159
160 // Grounded controls
161 if (grounded)
162 {
163 // Lock camera for short period when transitioning moving & standing still
164 lockCameraTimer += Time.deltaTime;
165 if (isMoving != wasMoving)
166 lockCameraTimer = 0.0f;
167
168 // We store speed and direction seperately,
169 // so that when the character stands still we still have a valid forward direction
170 // moveDirection is always normalized, and we only update it if there is user input.
171 if (targetDirection != Vector3.zero)
172 {
173 // If we are really slow, just snap to the target direction
174 if (moveSpeed < walkSpeed * 0.9f && grounded)
175 {
176 moveDirection = targetDirection.normalized;
177 }
178 // Otherwise smoothly turn towards it
179 else
180 {
181 moveDirection = Vector3.RotateTowards(moveDirection, targetDirection, rotateSpeed * Mathf.Deg2Rad * Time.deltaTime, 1000);
182
183 moveDirection = moveDirection.normalized;
184 }
185 }
186
187 // Smooth the speed based on the current target direction
188 float curSmooth = speedSmoothing * Time.deltaTime;
189
190 // Choose target speed
191 //* We want to support analog input but make sure you cant walk faster diagonally than just forward or sideways
192 float targetSpeed = Mathf.Min(targetDirection.magnitude, 1.0f);
193
194 _characterState = CharacterState.Idle;
195
196 // Pick speed modifier
197 if (Input.GetKey(KeyCode.LeftShift) | Input.GetKey(KeyCode.RightShift))
198 {
199 targetSpeed *= runSpeed;
200 _characterState = CharacterState.Running;
201 }
202 else if (Time.time - trotAfterSeconds > walkTimeStart)
203 {
204 targetSpeed *= trotSpeed;
205 _characterState = CharacterState.Trotting;
206 }
207 else
208 {
209 targetSpeed *= walkSpeed;
210 _characterState = CharacterState.Walking;
211 }
212
213 moveSpeed = Mathf.Lerp(moveSpeed, targetSpeed, curSmooth);
214
215 // Reset walk time start when we slow down
216 if (moveSpeed < walkSpeed * 0.3f)
217 walkTimeStart = Time.time;
218 }
219 // In air controls
220 else
221 {
222 // Lock camera while in air
223 if (jumping)
224 lockCameraTimer = 0.0f;
225
226 if (isMoving)
227 inAirVelocity += targetDirection.normalized * Time.deltaTime * inAirControlAcceleration;
228 }
229
230
231
232 }
File name: ThirdPersonController.cs
Copy
292 void Update()
293 {
294 if (isControllable)
295 {
296 if (Input.GetButtonDown("Jump"))
297 {
298 lastJumpButtonTime = Time.time;
299 }
300
301 UpdateSmoothedMovementDirection();
302
303 // Apply gravity
304 // - extra power jump modifies gravity
305 // - controlledDescent mode modifies gravity
306 ApplyGravity();
307
308 // Apply jumping logic
309 ApplyJumping();
310
311
312 // Calculate actual motion
313 Vector3 movement = moveDirection * moveSpeed + new Vector3(0, verticalSpeed, 0) + inAirVelocity;
314 movement *= Time.deltaTime;
315
316 // Move the controller
317 CharacterController controller = GetComponent
318 collisionFlags = controller.Move(movement);
319 }
320 velocity = (transform.position - lastPos)*25;
321
322 // ANIMATION sector
323 if (_animation)
324 {
325 if (_characterState == CharacterState.Jumping)
326 {
327 if (!jumpingReachedApex)
328 {
329 _animation[jumpPoseAnimation.name].speed = jumpAnimationSpeed;
330 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
331 _animation.CrossFade(jumpPoseAnimation.name);
332 }
333 else
334 {
335 _animation[jumpPoseAnimation.name].speed = -landAnimationSpeed;
336 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
337 _animation.CrossFade(jumpPoseAnimation.name);
338 }
339 }
340 else
341 {
342 if (this.isControllable && velocity.sqrMagnitude < 0.001f)
343 {
344 _characterState = CharacterState.Idle;
345 _animation.CrossFade(idleAnimation.name);
346 }
347 else
348 {
349 if (_characterState == CharacterState.Idle)
350 {
351 _animation.CrossFade(idleAnimation.name);
352 }
353 else if (_characterState == CharacterState.Running)
354 {
355 _animation[runAnimation.name].speed = runMaxAnimationSpeed;
356 if (this.isControllable)
357 {
358 _animation[runAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, runMaxAnimationSpeed);
359 }
360 _animation.CrossFade(runAnimation.name);
361 }
362 else if (_characterState == CharacterState.Trotting)
363 {
364 _animation[walkAnimation.name].speed = trotMaxAnimationSpeed;
365 if (this.isControllable)
366 {
367 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, trotMaxAnimationSpeed);
368 }
369 _animation.CrossFade(walkAnimation.name);
370 }
371 else if (_characterState == CharacterState.Walking)
372 {
373 _animation[walkAnimation.name].speed = walkMaxAnimationSpeed;
374 if (this.isControllable)
375 {
376 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, walkMaxAnimationSpeed);
377 }
378 _animation.CrossFade(walkAnimation.name);
379 }
380
381 }
382 }
383 }
384 // ANIMATION sector
385
386 // Set rotation to the move direction
387 if (IsGrounded())
388 {
389
390 transform.rotation = Quaternion.LookRotation(moveDirection);
391
392 }
393 else
394 {
395 /* This causes choppy behaviour when colliding with SIDES
396 * Vector3 xzMove = velocity;
397 xzMove.y = 0;
398 if (xzMove.sqrMagnitude > 0.001f)
399 {
400 transform.rotation = Quaternion.LookRotation(xzMove);
401 }*/
402 }
403
404 // We are in jump mode but just became grounded
405 if (IsGrounded())
406 {
407 lastGroundedTime = Time.time;
408 inAirVelocity = Vector3.zero;
409 if (jumping)
410 {
411 jumping = false;
412 SendMessage("DidLand", SendMessageOptions.DontRequireReceiver);
413 }
414 }
415
416 lastPos = transform.position;
417 }
File name: ThirdPersonController.cs
Copy
436 public bool IsGrounded()
437 {
438 return (collisionFlags & CollisionFlags.CollidedBelow) != 0;
439 }
File name: ThirdPersonController.cs
Copy
466 public bool IsGroundedWithTimeout()
467 {
468 return lastGroundedTime + groundedTimeout > Time.time;
469 }
Download file with original file name:Round
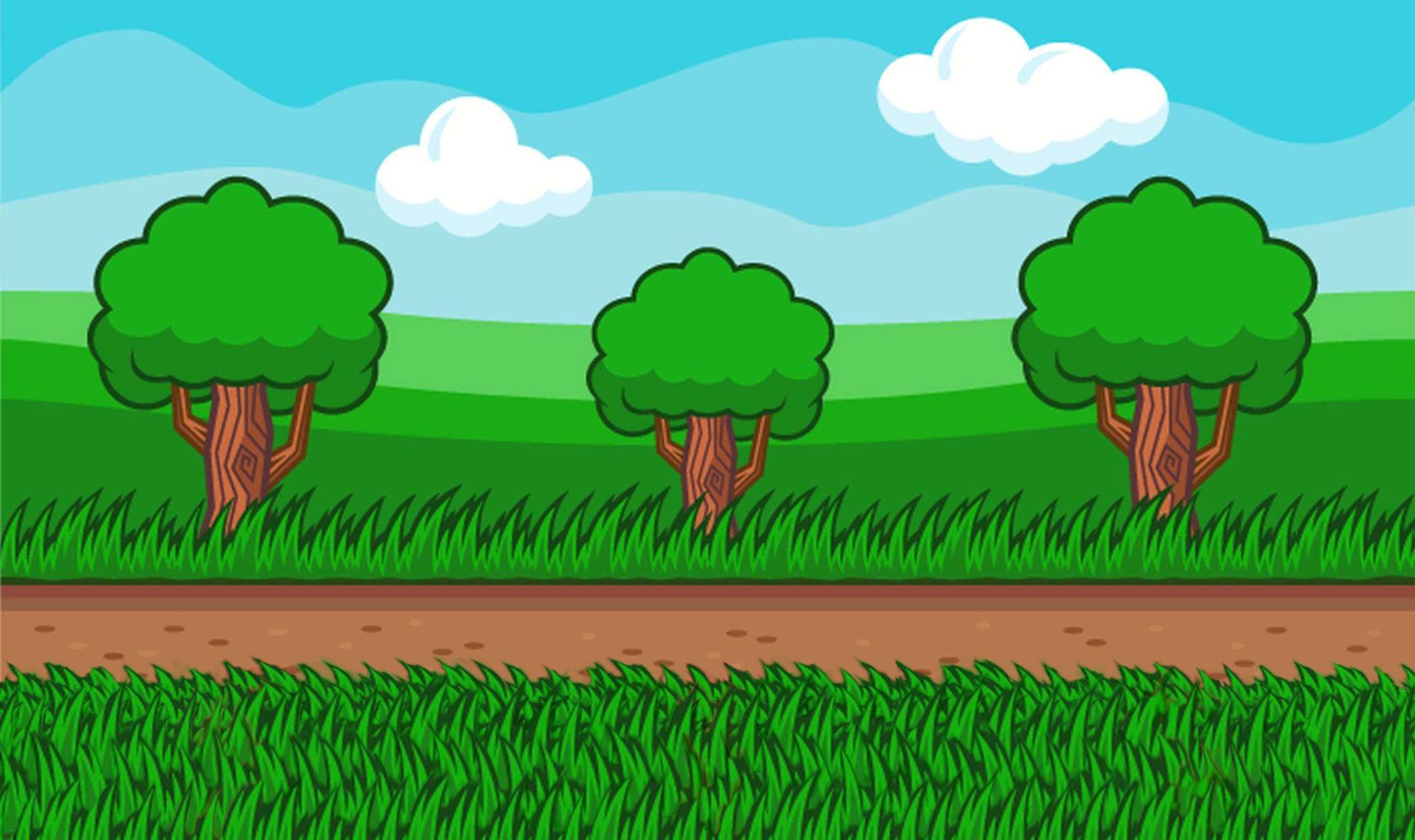
Round 161 lượt xem
Gõ tìm kiếm nhanh...