Won
How do I use Won
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: PhotonEditor.cs
Copy
390 protected virtual void OnGUI()
391 {
392 PhotonSetupStates oldGuiState = this.photonSetupState; // used to fix an annoying Editor input field issue: wont refresh until focus is changed.
393
394 GUI.SetNextControlName("");
395 this.scrollPos = GUILayout.BeginScrollView(this.scrollPos);
396
397 if (this.guiState == GUIState.Uninitialized)
398 {
399 this.ReApplySettingsToWindow();
400 this.guiState = (PhotonEditor.Current.HostType == ServerSettings.HostingOption.NotSet) ? GUIState.Setup : GUIState.Main;
401 }
402
403 if (this.guiState == GUIState.Main)
404 {
405 this.OnGuiMainWizard();
406 }
407 else
408 {
409 this.OnGuiRegisterCloudApp();
410 }
411
412 GUILayout.EndScrollView();
413
414 if (oldGuiState != this.photonSetupState)
415 {
416 GUI.FocusControl("");
417 }
418 }
File name: PhotonHandler.cs
Copy
232 internal IEnumerator PingAvailableRegionsCoroutine(bool connectToBest)
233 {
234 BestRegionCodeCurrently = CloudRegionCode.none;
235 while (PhotonNetwork.networkingPeer.AvailableRegions == null)
236 {
237 if (PhotonNetwork.connectionStateDetailed != PeerState.ConnectingToNameServer && PhotonNetwork.connectionStateDetailed != PeerState.ConnectedToNameServer)
238 {
239 Debug.LogError("Call ConnectToNameServer to ping available regions.");
240 yield break; // break if we don't connect to the nameserver at all
241 }
242
243 Debug.Log("Waiting for AvailableRegions. State: " + PhotonNetwork.connectionStateDetailed + " Server: " + PhotonNetwork.Server + " PhotonNetwork.networkingPeer.AvailableRegions " + (PhotonNetwork.networkingPeer.AvailableRegions != null));
244 yield return new WaitForSeconds(0.25f); // wait until pinging finished (offline mode won't ping)
245 }
246
247 if (PhotonNetwork.networkingPeer.AvailableRegions == null || PhotonNetwork.networkingPeer.AvailableRegions.Count == 0)
248 {
249 Debug.LogError("No regions available. Are you sure your appid is valid and setup?");
250 yield break; // break if we don't get regions at all
251 }
252
253 //#if !UNITY_EDITOR && (UNITY_ANDROID || UNITY_IPHONE)
254 //#pragma warning disable 0162 // the library variant defines if we should use PUN's SocketUdp variant (at all)
255 //if (PhotonPeer.NoSocket)
256 //{
257 // if (PhotonNetwork.logLevel >= PhotonLogLevel.Informational)
258 // {
259 // Debug.Log("PUN disconnects to re-use native sockets for pining servers and to find the best.");
260 // }
261 // PhotonNetwork.Disconnect();
262 //}
263 //#pragma warning restore 0162
264 //#endif
265
266 PhotonPingManager pingManager = new PhotonPingManager();
267 foreach (Region region in PhotonNetwork.networkingPeer.AvailableRegions)
268 {
269 SP.StartCoroutine(pingManager.PingSocket(region));
270 }
271
272 while (!pingManager.Done)
273 {
274 yield return new WaitForSeconds(0.1f); // wait until pinging finished (offline mode won't ping)
275 }
276
277
278 Region best = pingManager.BestRegion;
279 PhotonHandler.BestRegionCodeCurrently = best.Code;
280 PhotonHandler.BestRegionCodeInPreferences = best.Code;
281
282 Debug.Log("Found best region: " + best.Code + " ping: " + best.Ping + ". Calling ConnectToRegionMaster() is: " + connectToBest);
283
284
285 if (connectToBest)
286 {
287 PhotonNetwork.networkingPeer.ConnectToRegionMaster(best.Code);
288 }
289 }
File name: PhotonStatsGui.cs
Copy
53 public void Update()
54 {
55 if (Input.GetKeyDown(KeyCode.Tab) && Input.GetKey(KeyCode.LeftShift))
56 {
57 this.statsWindowOn = !this.statsWindowOn;
58 this.statsOn = true; // enable stats when showing the window
59 }
60 }
File name: PhotonStatsGui.cs
Copy
62 public void OnGUI()
63 {
64 if (PhotonNetwork.networkingPeer.TrafficStatsEnabled != statsOn)
65 {
66 PhotonNetwork.networkingPeer.TrafficStatsEnabled = this.statsOn;
67 }
68
69 if (!this.statsWindowOn)
70 {
71 return;
72 }
73
74 this.statsRect = GUILayout.Window(this.WindowId, this.statsRect, this.TrafficStatsWindow, "Messages (shift+tab)");
75 }
File name: SmoothSyncMovement.cs
Copy
7 public void Awake()
8 {
9 if (this.photonView == null || this.photonView.observed != this)
10 {
11 Debug.LogWarning(this + " is not observed by this object's photonView! OnPhotonSerializeView() in this class won't be used.");
12 }
13 }
File name: Board.cs
Copy
69 public bool HasWon(Seed seed)
70 {
71 if (lastChangedCell == null)
72 {
73 return false;
74 }
75
76 return HasWonInTheRow(seed)
77 || HasWonInTheColumn(seed)
78 || HasWonInTheDiagonal(seed)
79 || HasWonInTheOppositeDiagonal(seed);
80 }
File name: Board.cs
Copy
82 private bool HasWonInTheRow(Seed seed)
83 {
84 int row = lastChangedCell.Row;
85
86 return cells[row, 0].HasSeed(seed)
87 && cells[row, 1].HasSeed(seed)
88 && cells[row, 2].HasSeed(seed);
89 }
File name: Board.cs
Copy
91 private bool HasWonInTheColumn(Seed seed)
92 {
93 int col = lastChangedCell.Col;
94
95 return cells[0, col].HasSeed(seed)
96 && cells[1, col].HasSeed(seed)
97 && cells[2, col].HasSeed(seed);
98 }
File name: Board.cs
Copy
100 private bool HasWonInTheDiagonal(Seed seed)
101 {
102 return cells[0, 0].HasSeed(seed)
103 && cells[1, 1].HasSeed(seed)
104 && cells[2, 2].HasSeed(seed);
105 }
File name: Board.cs
Copy
107 private bool HasWonInTheOppositeDiagonal(Seed seed)
108 {
109 return cells[0, 2].HasSeed(seed)
110 && cells[1, 1].HasSeed(seed)
111 && cells[2, 0].HasSeed(seed);
112 }
Download file with original file name:Won
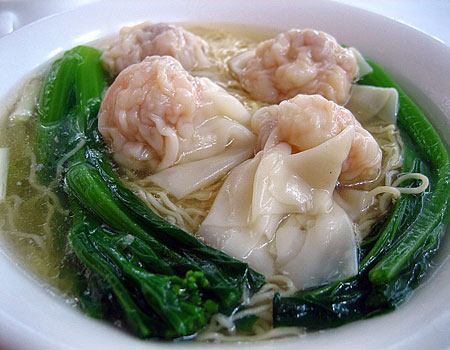
Won 127 lượt xem
Gõ tìm kiếm nhanh...