Look
How do I use Look
Below are practical examples compiled from projects for learning and reference purposes
Featured Snippets
File name: MoveCam.cs
Copy
22 private void Update()
23 {
24 camTransform.position = Vector3.Slerp(camTransform.position, randomPos, Time.deltaTime);
25 camTransform.LookAt(lookAt);
26 if (Vector3.Distance(camTransform.position, randomPos) < 0.5f)
27 {
28 randomPos = originalPos + new Vector3(Random.Range(-2, 2), Random.Range(-2, 2), Random.Range(-1, 1));
29 }
30 }
File name: PickupCamera.cs
Copy
198 void SetUpRotation( Vector3 centerPos, Vector3 headPos )
199 {
200 // Now it's getting hairy. The devil is in the details here, the big issue is jumping of course.
201 // * When jumping up and down we don't want to center the guy in screen space.
202 // This is important to give a feel for how high you jump and avoiding large camera movements.
203 //
204 // * At the same time we dont want him to ever go out of screen and we want all rotations to be totally smooth.
205 //
206 // So here is what we will do:
207 //
208 // 1. We first find the rotation around the y axis. Thus he is always centered on the y-axis
209 // 2. When grounded we make him be centered
210 // 3. When jumping we keep the camera rotation but rotate the camera to get him back into view if his head is above some threshold
211 // 4. When landing we smoothly interpolate towards centering him on screen
212 Vector3 cameraPos = cameraTransform.position;
213 Vector3 offsetToCenter = centerPos - cameraPos;
214
215 // Generate base rotation only around y-axis
216 Quaternion yRotation = Quaternion.LookRotation( new Vector3( offsetToCenter.x, 0, offsetToCenter.z ) );
217
218 Vector3 relativeOffset = Vector3.forward * distance + Vector3.down * height;
219 cameraTransform.rotation = yRotation * Quaternion.LookRotation( relativeOffset );
220
221 // Calculate the projected center position and top position in world space
222 Ray centerRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, 0.5f, 1 ) );
223 Ray topRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, clampHeadPositionScreenSpace, 1 ) );
224
225 Vector3 centerRayPos = centerRay.GetPoint( distance );
226 Vector3 topRayPos = topRay.GetPoint( distance );
227
228 float centerToTopAngle = Vector3.Angle( centerRay.direction, topRay.direction );
229
230 float heightToAngle = centerToTopAngle / ( centerRayPos.y - topRayPos.y );
231
232 float extraLookAngle = heightToAngle * ( centerRayPos.y - centerPos.y );
233 if( extraLookAngle < centerToTopAngle )
234 {
235 extraLookAngle = 0;
236 }
237 else
238 {
239 extraLookAngle = extraLookAngle - centerToTopAngle;
240 cameraTransform.rotation *= Quaternion.Euler( -extraLookAngle, 0, 0 );
241 }
242 }
File name: PickupController.cs
Copy
73 // Are we jumping? (Initiated with jump button and not grounded yet)
77 // Are we moving backwards (This locks the camera to not do a 180 degree spin)
81 // When did the user start walking (Used for going into trot after a while)
87 // the height we jumped from (Used to determine for how long to apply extra jump power after jumping.)
101 void Awake()
102 {
103 // PUN: automatically determine isControllable, if this GO has a PhotonView
104 PhotonView pv = this.gameObject.GetComponent
105 if (pv != null)
106 {
107 isControllable = pv.isMine;
108
109 // The pickup demo assigns this GameObject as the PhotonPlayer.TagObject. This way, we can access this character (controller, position, etc) easily
110 if (this.AssignAsTagObject)
111 {
112 pv.owner.TagObject = this.gameObject;
113 }
114
115 // please note: we change this setting on ANY PickupController if "DoRotate" is off. not only locally when it's "our" GameObject!
116 if (pv.observed is Transform && !DoRotate)
117 {
118 pv.onSerializeTransformOption = OnSerializeTransform.OnlyPosition;
119 }
120 }
121
122
123 moveDirection = transform.TransformDirection(Vector3.forward);
124
125 _animation = GetComponent
126 if (!_animation)
127 Debug.Log("The character you would like to control doesn't have animations. Moving her might look weird.");
128
129 if (!idleAnimation)
130 {
131 _animation = null;
132 Debug.Log("No idle animation found. Turning off animations.");
133 }
134 if (!walkAnimation)
135 {
136 _animation = null;
137 Debug.Log("No walk animation found. Turning off animations.");
138 }
139 if (!runAnimation)
140 {
141 _animation = null;
142 Debug.Log("No run animation found. Turning off animations.");
143 }
144 if (!jumpPoseAnimation && canJump)
145 {
146 _animation = null;
147 Debug.Log("No jump animation found and the character has canJump enabled. Turning off animations.");
148 }
149 }
File name: PickupController.cs
Copy
151 void Update()
152 {
153 if (isControllable)
154 {
155 if (Input.GetButtonDown("Jump"))
156 {
157 lastJumpButtonTime = Time.time;
158 }
159
160 UpdateSmoothedMovementDirection();
161
162 // Apply gravity
163 // - extra power jump modifies gravity
164 // - controlledDescent mode modifies gravity
165 ApplyGravity();
166
167 // Apply jumping logic
168 ApplyJumping();
169
170
171 // Calculate actual motion
172 Vector3 movement = moveDirection * moveSpeed + new Vector3(0, verticalSpeed, 0) + inAirVelocity;
173 movement *= Time.deltaTime;
174
175 //Debug.Log(movement.x.ToString("0.000") + ":" + movement.z.ToString("0.000"));
176
177 // Move the controller
178 CharacterController controller = GetComponent
179 collisionFlags = controller.Move(movement);
180
181 }
182
183 // PUN: if a remote position is known, we smooth-move to it (being late(r) but smoother)
184 if (this.remotePosition != Vector3.zero)
185 {
186 transform.position = Vector3.Lerp(transform.position, this.remotePosition, Time.deltaTime * this.RemoteSmoothing);
187 }
188
189 velocity = (transform.position - lastPos)*25;
190
191 // ANIMATION sector
192 if (_animation)
193 {
194 if (_characterState == PickupCharacterState.Jumping)
195 {
196 if (!jumpingReachedApex)
197 {
198 _animation[jumpPoseAnimation.name].speed = jumpAnimationSpeed;
199 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
200 _animation.CrossFade(jumpPoseAnimation.name);
201 }
202 else
203 {
204 _animation[jumpPoseAnimation.name].speed = -landAnimationSpeed;
205 _animation[jumpPoseAnimation.name].wrapMode = WrapMode.ClampForever;
206 _animation.CrossFade(jumpPoseAnimation.name);
207 }
208 }
209 else
210 {
211 if (_characterState == PickupCharacterState.Idle)
212 {
213 _animation.CrossFade(idleAnimation.name);
214 }
215 else if (_characterState == PickupCharacterState.Running)
216 {
217 _animation[runAnimation.name].speed = runMaxAnimationSpeed;
218 if (this.isControllable)
219 {
220 _animation[runAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, runMaxAnimationSpeed);
221 }
222 _animation.CrossFade(runAnimation.name);
223 }
224 else if (_characterState == PickupCharacterState.Trotting)
225 {
226 _animation[walkAnimation.name].speed = trotMaxAnimationSpeed;
227 if (this.isControllable)
228 {
229 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, trotMaxAnimationSpeed);
230 }
231 _animation.CrossFade(walkAnimation.name);
232 }
233 else if (_characterState == PickupCharacterState.Walking)
234 {
235 _animation[walkAnimation.name].speed = walkMaxAnimationSpeed;
236 if (this.isControllable)
237 {
238 _animation[walkAnimation.name].speed = Mathf.Clamp(velocity.magnitude, 0.0f, walkMaxAnimationSpeed);
239 }
240 _animation.CrossFade(walkAnimation.name);
241 }
242
243 if (_characterState != PickupCharacterState.Running)
244 {
245 _animation[runAnimation.name].time = 0.0f;
246 }
247 }
248 }
249 // ANIMATION sector
250
251 // Set rotation to the move direction
252 if (IsGrounded())
253 {
254 // a specialty of this controller: you can disable rotation!
255 if (DoRotate)
256 {
257 transform.rotation = Quaternion.LookRotation(moveDirection);
258 }
259 }
260 else
261 {
262 /* This causes choppy behaviour when colliding with SIDES
263 * Vector3 xzMove = velocity;
264 xzMove.y = 0;
265 if (xzMove.sqrMagnitude > 0.001f)
266 {
267 transform.rotation = Quaternion.LookRotation(xzMove);
268 }*/
269 }
270
271 // We are in jump mode but just became grounded
272 if (IsGrounded())
273 {
274 lastGroundedTime = Time.time;
275 inAirVelocity = Vector3.zero;
276 if (jumping)
277 {
278 jumping = false;
279 SendMessage("DidLand", SendMessageOptions.DontRequireReceiver);
280 }
281 }
282
283 lastPos = transform.position;
284 }
File name: RPGCamera.cs
Copy
20 void Start()
21 {
22 m_CameraTransform = transform.GetChild( 0 );
23 m_LocalForwardVector = m_CameraTransform.forward;
24
25 m_Distance = -m_CameraTransform.localPosition.z / m_CameraTransform.forward.z;
26 m_Distance = Mathf.Clamp( m_Distance, MinimumDistance, MaximumDistance );
27 m_LookAtPoint = m_CameraTransform.localPosition + m_LocalForwardVector * m_Distance;
28 }
File name: RPGCamera.cs
Copy
43 void UpdateZoom()
44 {
45 m_CameraTransform.localPosition = m_LookAtPoint - m_LocalForwardVector * m_Distance;
46 }
File name: ThirdPersonCamera.cs
Copy
190 void SetUpRotation( Vector3 centerPos, Vector3 headPos )
191 {
192 // Now it's getting hairy. The devil is in the details here, the big issue is jumping of course.
193 // * When jumping up and down we don't want to center the guy in screen space.
194 // This is important to give a feel for how high you jump and avoiding large camera movements.
195 //
196 // * At the same time we dont want him to ever go out of screen and we want all rotations to be totally smooth.
197 //
198 // So here is what we will do:
199 //
200 // 1. We first find the rotation around the y axis. Thus he is always centered on the y-axis
201 // 2. When grounded we make him be centered
202 // 3. When jumping we keep the camera rotation but rotate the camera to get him back into view if his head is above some threshold
203 // 4. When landing we smoothly interpolate towards centering him on screen
204 Vector3 cameraPos = cameraTransform.position;
205 Vector3 offsetToCenter = centerPos - cameraPos;
206
207 // Generate base rotation only around y-axis
208 Quaternion yRotation = Quaternion.LookRotation( new Vector3( offsetToCenter.x, 0, offsetToCenter.z ) );
209
210 Vector3 relativeOffset = Vector3.forward * distance + Vector3.down * height;
211 cameraTransform.rotation = yRotation * Quaternion.LookRotation( relativeOffset );
212
213 // Calculate the projected center position and top position in world space
214 Ray centerRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, 0.5f, 1 ) );
215 Ray topRay = m_CameraTransformCamera.ViewportPointToRay( new Vector3( 0.5f, clampHeadPositionScreenSpace, 1 ) );
216
217 Vector3 centerRayPos = centerRay.GetPoint( distance );
218 Vector3 topRayPos = topRay.GetPoint( distance );
219
220 float centerToTopAngle = Vector3.Angle( centerRay.direction, topRay.direction );
221
222 float heightToAngle = centerToTopAngle / ( centerRayPos.y - topRayPos.y );
223
224 float extraLookAngle = heightToAngle * ( centerRayPos.y - centerPos.y );
225 if( extraLookAngle < centerToTopAngle )
226 {
227 extraLookAngle = 0;
228 }
229 else
230 {
231 extraLookAngle = extraLookAngle - centerToTopAngle;
232 cameraTransform.rotation *= Quaternion.Euler( -extraLookAngle, 0, 0 );
233 }
234 }
File name: ThirdPersonController.cs
Copy
71 // Are we jumping? (Initiated with jump button and not grounded yet)
75 // Are we moving backwards (This locks the camera to not do a 180 degree spin)
79 // When did the user start walking (Used for going into trot after a while)
85 // the height we jumped from (Used to determine for how long to apply extra jump power after jumping.)
92 void Awake()
93 {
94 moveDirection = transform.TransformDirection(Vector3.forward);
95
96 _animation = GetComponent
97 if (!_animation)
98 Debug.Log("The character you would like to control doesn't have animations. Moving her might look weird.");
99
100 /*
101 public AnimationClip idleAnimation;
102 public AnimationClip walkAnimation;
103 public AnimationClip runAnimation;
104 public AnimationClip jumpPoseAnimation;
105 */
106 if (!idleAnimation)
107 {
108 _animation = null;
109 Debug.Log("No idle animation found. Turning off animations.");
110 }
111 if (!walkAnimation)
112 {
113 _animation = null;
114 Debug.Log("No walk animation found. Turning off animations.");
115 }
116 if (!runAnimation)
117 {
118 _animation = null;
119 Debug.Log("No run animation found. Turning off animations.");
120 }
121 if (!jumpPoseAnimation && canJump)
122 {
123 _animation = null;
124 Debug.Log("No jump animation found and the character has canJump enabled. Turning off animations.");
125 }
126
127 }
File name: PhotonViewInspector.cs
Copy
21 public override void OnInspectorGUI()
22 {
23 #if UNITY_3_5
24 EditorGUIUtility.LookLikeInspector();
25 #endif
26 //EditorGUI.indentLevel = 1;
27
28 m_Target = (PhotonView)this.target;
29 bool isProjectPrefab = EditorUtility.IsPersistent(m_Target.gameObject);
30
31 if( m_Target.ObservedComponents == null )
32 {
33 m_Target.ObservedComponents = new System.Collections.Generic.List
34 }
35
36 if( m_Target.ObservedComponents.Count == 0 )
37 {
38 m_Target.ObservedComponents.Add( null );
39 }
40
41 EditorGUILayout.BeginHorizontal();
42 // Owner
43 if (isProjectPrefab)
44 {
45 EditorGUILayout.LabelField("Owner:", "Set at runtime");
46 }
47 else if (m_Target.isSceneView)
48 {
49 EditorGUILayout.LabelField("Owner", "Scene");
50 }
51 else
52 {
53 PhotonPlayer owner = m_Target.owner;
54 string ownerInfo = (owner != null) ? owner.name : "
55
56 if (string.IsNullOrEmpty(ownerInfo))
57 {
58 ownerInfo = "
59 }
60
61 EditorGUILayout.LabelField("Owner", "[" + m_Target.ownerId + "] " + ownerInfo);
62 }
63
64 // ownership requests
65 EditorGUI.BeginDisabledGroup(Application.isPlaying);
66 m_Target.ownershipTransfer = (OwnershipOption)EditorGUILayout.EnumPopup(m_Target.ownershipTransfer, GUILayout.Width(100));
67 EditorGUI.EndDisabledGroup();
68
69 EditorGUILayout.EndHorizontal();
70
71
72 // View ID
73 if (isProjectPrefab)
74 {
75 EditorGUILayout.LabelField("View ID", "Set at runtime");
76 }
77 else if (EditorApplication.isPlaying)
78 {
79 EditorGUILayout.LabelField("View ID", m_Target.viewID.ToString());
80 }
81 else
82 {
83 int idValue = EditorGUILayout.IntField("View ID [1.."+(PhotonNetwork.MAX_VIEW_IDS-1)+"]", m_Target.viewID);
84 m_Target.viewID = idValue;
85 }
86
87
88
89 // Locally Controlled
90 if (EditorApplication.isPlaying)
91 {
92 string masterClientHint = PhotonNetwork.isMasterClient ? "(master)" : "";
93 EditorGUILayout.Toggle("Controlled locally: " + masterClientHint, m_Target.isMine);
94 }
95
96
97
98 //DrawOldObservedItem();
99 ConvertOldObservedItemToObservedList();
100
101
102 // ViewSynchronization (reliability)
103 if (m_Target.synchronization == ViewSynchronization.Off)
104 {
105 GUI.color = Color.grey;
106 }
107
108 EditorGUILayout.PropertyField( serializedObject.FindProperty( "synchronization" ), new GUIContent( "Observe option:" ) );
109
110 if( m_Target.synchronization != ViewSynchronization.Off &&
111 m_Target.ObservedComponents.FindAll( item => item != null ).Count == 0 )
112 {
113 GUILayout.BeginVertical( GUI.skin.box );
114 GUILayout.Label( "Warning", EditorStyles.boldLabel );
115 GUILayout.Label( "Setting the synchronization option only makes sense if you observe something." );
116 GUILayout.EndVertical();
117 }
118
119 /*ViewSynchronization vsValue = (ViewSynchronization)EditorGUILayout.EnumPopup("Observe option:", m_Target.synchronization);
120 if (vsValue != m_Target.synchronization)
121 {
122 m_Target.synchronization = vsValue;
123 if (m_Target.synchronization != ViewSynchronization.Off && m_Target.observed == null)
124 {
125 EditorUtility.DisplayDialog("Warning", "Setting the synchronization option only makes sense if you observe something.", "OK, I will fix it.");
126 }
127 }*/
128
129 DrawSpecificTypeSerializationOptions();
130
131 GUI.color = Color.white;
132 DrawObservedComponentsList();
133
134 // Cleanup: save and fix look
135 if (GUI.changed)
136 {
137 EditorUtility.SetDirty(m_Target);
138 PhotonViewHandler.HierarchyChange(); // TODO: check if needed
139 }
140
141 GUI.color = Color.white;
142 EditorGUIUtility.LookLikeControls();
143 }
File name: ServerSettingsInspector.cs
Copy
17 public override void OnInspectorGUI()
18 {
19 ServerSettings settings = (ServerSettings)this.target;
20
21 #if UNITY_3_5
22 EditorGUIUtility.LookLikeInspector();
23 #endif
24
25
26 settings.HostType = (ServerSettings.HostingOption)EditorGUILayout.EnumPopup("Hosting", settings.HostType);
27 EditorGUI.indentLevel = 1;
28
29 switch (settings.HostType)
30 {
31 case ServerSettings.HostingOption.BestRegion:
32 case ServerSettings.HostingOption.PhotonCloud:
33 if (settings.HostType == ServerSettings.HostingOption.PhotonCloud)
34 settings.PreferredRegion = (CloudRegionCode)EditorGUILayout.EnumPopup("Region", settings.PreferredRegion);
35 settings.AppID = EditorGUILayout.TextField("AppId", settings.AppID);
36 settings.Protocol = (ConnectionProtocol)EditorGUILayout.EnumPopup("Protocol", settings.Protocol);
37
38 if (string.IsNullOrEmpty(settings.AppID) || settings.AppID.Equals("master"))
39 {
40 EditorGUILayout.HelpBox("The Photon Cloud needs an AppId (GUID) set.\nYou can find it online in your Dashboard.", MessageType.Warning);
41 }
42 break;
43
44 case ServerSettings.HostingOption.SelfHosted:
45 bool hidePort = false;
46 if (settings.Protocol == ConnectionProtocol.Udp && (settings.ServerPort == 4530 || settings.ServerPort == 0))
47 {
48 settings.ServerPort = 5055;
49 }
50 else if (settings.Protocol == ConnectionProtocol.Tcp && (settings.ServerPort == 5055 || settings.ServerPort == 0))
51 {
52 settings.ServerPort = 4530;
53 }
54 #if RHTTP
55 if (settings.Protocol == ConnectionProtocol.RHttp)
56 {
57 settings.ServerPort = 0;
58 hidePort = true;
59 }
60 #endif
61 settings.ServerAddress = EditorGUILayout.TextField("Server Address", settings.ServerAddress);
62 settings.ServerAddress = settings.ServerAddress.Trim();
63 if (!hidePort)
64 {
65 settings.ServerPort = EditorGUILayout.IntField("Server Port", settings.ServerPort);
66 }
67 settings.Protocol = (ConnectionProtocol)EditorGUILayout.EnumPopup("Protocol", settings.Protocol);
68 settings.AppID = EditorGUILayout.TextField("AppId", settings.AppID);
69 break;
70
71 case ServerSettings.HostingOption.OfflineMode:
72 EditorGUI.indentLevel = 0;
73 EditorGUILayout.HelpBox("In 'Offline Mode', the client does not communicate with a server.\nAll settings are hidden currently.", MessageType.Info);
74 break;
75
76 case ServerSettings.HostingOption.NotSet:
77 EditorGUI.indentLevel = 0;
78 EditorGUILayout.HelpBox("Hosting is 'Not Set'.\nConnectUsingSettings() will not be able to connect.\nSelect another option or run the PUN Wizard.", MessageType.Info);
79 break;
80
81 default:
82 DrawDefaultInspector();
83 break;
84 }
85
86 if (PhotonEditor.CheckPunPlus())
87 {
88 settings.Protocol = ConnectionProtocol.Udp;
89 EditorGUILayout.HelpBox("You seem to use PUN+.\nPUN+ only supports reliable UDP so the protocol is locked.", MessageType.Info);
90 }
91
92 settings.AppID = settings.AppID.Trim();
93
94 EditorGUI.indentLevel = 0;
95 SerializedObject sObj = new SerializedObject(this.target);
96 SerializedProperty sRpcs = sObj.FindProperty("RpcList");
97 EditorGUILayout.PropertyField(sRpcs, true);
98 sObj.ApplyModifiedProperties();
99
100 GUILayout.BeginHorizontal();
101 GUILayout.Space(20);
102 if (GUILayout.Button("Refresh RPCs"))
103 {
104 PhotonEditor.UpdateRpcList();
105 Repaint();
106 }
107 if (GUILayout.Button("Clear RPCs"))
108 {
109 PhotonEditor.ClearRpcList();
110 }
111 if (GUILayout.Button("Log HashCode"))
112 {
113 Debug.Log("RPC-List HashCode: " + RpcListHashCode() + ". Make sure clients that send each other RPCs have the same RPC-List.");
114 }
115 GUILayout.Space(20);
116 GUILayout.EndHorizontal();
117
118 //SerializedProperty sp = serializedObject.FindProperty("RpcList");
119 //EditorGUILayout.PropertyField(sp, true);
120
121 if (GUI.changed)
122 {
123 EditorUtility.SetDirty(target);
124 }
125 }
Download file with original file name:Look
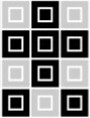
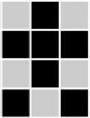
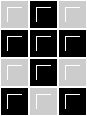


Look 108 lượt xem
Gõ tìm kiếm nhanh...