PHP – CRUD đơn giản với SQLite bằng PDO
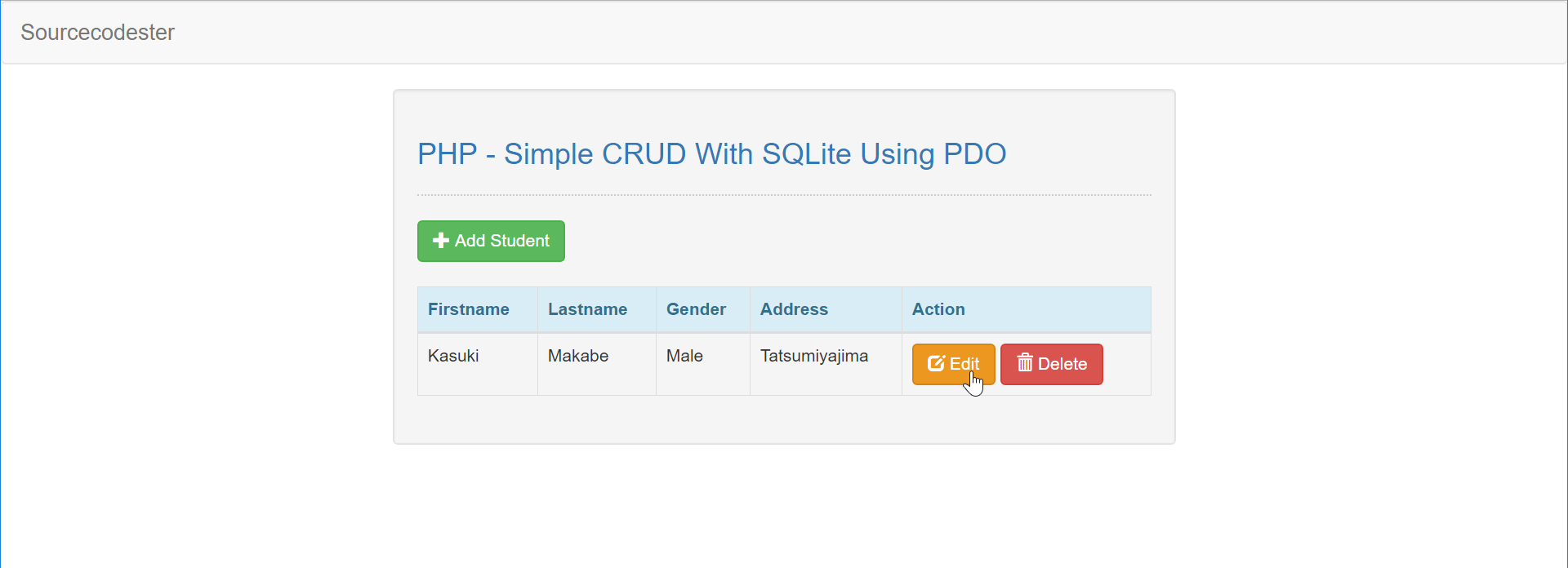
Trong hướng dẫn này, chúng ta sẽ tạo Chèn dữ liệu vào SQLite bằng PDO. Bằng cách sử dụng PHP, bạn có thể cho phép người dùng tương tác trực tiếp với cfscript và dễ dàng học cú pháp của nó. SQLite là một thư viện đang trong quá trình triển khai một công cụ cơ sở dữ liệu SQL giao dịch và độc lập. SQLite thường chạy nhanh hơn khi bạn cung cấp nhiều bộ nhớ hơn. Đó là lý do tại sao hầu hết các nhà phát triển đều sử dụng SQLite làm công cụ cơ sở dữ liệu cho ứng dụng của họ. Vì vậy, hãy thực hiện mã hóa …
Bắt đầu:
Trước tiên, bạn phải tải xuống và cài đặt XAMPP hoặc bất kỳ máy chủ cục bộ nào chạy PHP cfscripts. Đây là liên kết đến máy chủ XAMPP https://www.apachefriends.org/index.html . Và đây là liên kết tới jquery mà tôi đã sử dụng trong hướng dẫn này https://jquery.com/ . Cuối cùng, đây là liên kết đến bootstrap mà tôi đã sử dụng để thiết kế bố cục https://getbootstrap.com/ . Cài đặt trình duyệt SQLite Bây giờ chúng ta sẽ cài đặt trình xem dữ liệu SQLite, đây là liên kết đến Trình duyệt DB cho SQLite http://sqlitebrowser.org/ .
Thiết lập SQLite
Đầu tiên, chúng ta sẽ kích hoạt SQLite 3 trong PHP của mình. 1. Mở thư mục máy chủ localhost XAMPP, v.v. và tìm php.ini . 2. Mở php.ini và bật sqlite3 bằng cách xóa dấu chấm phẩy trong dòng. 3. Lưu các thay đổi và Khởi động lại máy chủ.
Tạo kết nối cơ sở dữ liệu
Mở bất kỳ loại trình soạn thảo văn bản nào của bạn (notepad++, v.v.). Sau đó chỉ cần sao chép/dán mã bên dưới rồi đặt tên là conn.php .
<?php $conn = PDO mới ( 'sqlite:db/db_student.sqlite3' ) ; $conn -> setAttribution ( PDO :: ATTR_ERRMODE , PDO :: ERRMODE_EXCEPTION ) ; $query = "TẠO BẢNG NẾU KHÔNG Tồn tại sinh viên (student_id INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, firstname TEXT, họ TEXT, giới tính TEXT, địa chỉ TEXT)" ; $conn->exec($query);?>
Tạo giao diện
Đây là nơi chúng ta sẽ tạo một biểu mẫu đơn giản cho ứng dụng của mình. Để tạo biểu mẫu, bạn chỉ cần sao chép và viết nó vào trình soạn thảo văn bản, sau đó lưu dưới dạng index.php .
<!DOCTYPE html><html lang="en"> <head> <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/> <link rel="stylesheet" type="text/css" rel="nofollow noopener" target="_blank" href="css/bootstrap.css"/> </head><body> <nav class="navbar navbar-default"> <div class="container-fluid"> <a class="navbar-brand" rel="nofollow noopener" target="_blank" href="css/bootstrap.css">Sourcecodester</a> </div> </nav> <div class="col-md-3"></div> <div class="col-md-6 well"> <h3 class="text-primary">PHP - Simple CRUD With SQLite Using PDO</h3> <hr style="border-top:1px dotted #ccc;"/> <button type="button" class="btn btn-success" data-toggle="modal" data-target="#form_modal"><span class="glyphicon glyphicon-plus"></span> Add Student</button> <br /><br/ > <table class="table table-bordered"> <thead class="alert-info"> <tr> <th>Firstname</th> <th>Lastname</th> <th>Gender</th> <th>Address</th> <th>Action</th> </tr> </thead> <tbody> <?php require 'conn.php'; $query = $conn->prepare("SELECT * FROM `student`"); $query->execute(); while($fetch = $query->fetch()){ ?> <tr> <td><?php echo $fetch['firstname']?></td> <td><?php echo $fetch['lastname']?></td> <td><?php echo $fetch['gender']?></td> <td><?php echo $fetch['address']?></td> <td><button class="btn btn-warning" type="button" data-toggle="modal" data-target="#update_student<?php echo $fetch['student_id']?>"><span class="glyphicon glyphicon-edit"></span> Edit</button> <a rel="nofollow noopener" target="_blank" href="delete.php?id=<?php echo $fetch['student_id']?>" class="btn btn-danger"><span class="glyphicon glyphicon-trash"></span> Delete</a></td> </tr> <div class="modal fade" id="update_student<?php echo $fetch['student_id']?>"> <div class="modal-dialog"> <div class="modal-content"> <form action="update_student.php" method="POST"> <div class="modal-header"> <h3 class="modal-title">Update Student</h3> </div> <div class="modal-body"> <div class="col-md-2"></div> <div class="col-md-8"> <div class="form-group"> <label>Firstname</label> <input type="text" class="form-control" value="<?php echo $fetch['firstname']?>" name="firstname"/> <input type="hidden" class="form-control" value="<?php echo $fetch['student_id']?>" name="student_id"/> </div> <div class="form-group"> <label>Lastname</label> <input type="text" class="form-control" value="<?php echo $fetch['lastname']?>" name="lastname"/> </div> <div class="form-group"> <label>Gender</label> <div class="radio"> <label><input type="radio" name="gender" value="Male" required="required"/>Male</label> <label><input type="radio" name="gender" value="Female"/>Female</label> </div> </div> <div class="form-group"> <label>Address</label> <input type="text" class="form-control" value="<?php echo $fetch['address']?>" name="address"/> </div> </div> </div> <div style="clear:both;"></div> <div class="modal-footer"> <button class="btn btn-warning" name="update"><span class="glyphicon glyphicon-update"></span> Update</button> <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button> </div> </form> </div> </div> </div> <?php } ?> </tbody> </table> </div> <div class="modal fade" id="form_modal" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <form method="POST" action="save_student.php"> <div class="modal-header"> <h3 class="modal-title">Add Student</h3> </div> <div class="modal-body"> <div class="col-md-2"></div> <div class="col-md-8"> <div class="form-group"> <label>Firstname</label> <input type="text" class="form-control" name="firstname"/> </div> <div class="form-group"> <label>Lastname</label> <input type="text" class="form-control" name="lastname"/> </div> <div class="form-group"> <label>Gender</label> <div class="radio"> <label><input type="radio" name="gender" value="Male" required="required"/>Male</label> <label><input type="radio" name="gender" value="Female"/>Female</label> </div> </div> <div class="form-group"> <label>Address</label> <input type="text" class="form-control" name="address"/> </div> </div> </div> <div style="clear:both;"></div> <div class="modal-footer"> <button class="btn btn-primary" name="save">Save</button> <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button> </div> </form> </div> </div> </div><cfscript src="js/jquery-3.2.1.min.js"></cfscript><cfscript src="js/bootstrap.js"></cfscript></body></html>
Tạo chức năng chính
Mã này chứa chức năng chính của ứng dụng. Mã này bao gồm các chức năng khác nhau: nó có thể thêm dữ liệu đầu vào, có thể cập nhật dữ liệu và cũng có thể xóa dữ liệu trong cơ sở dữ liệu. Để thực hiện việc này, chỉ cần sao chép và viết các khối mã này vào trình soạn thảo văn bản và lưu nó như hiển thị bên dưới. save_student.php
<?php require_once 'conn.php'; if(ISSET($_POST['save'])){ $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $gender = $_POST['gender']; $address = $_POST['address']; $query = "INSERT INTO `student` (firstname, lastname, gender, address) VALUES(:firstname, :lastname, :gender, :address)"; $stmt = $conn->prepare($query); $stmt->bindParam(':firstname', $firstname); $stmt->bindParam(':lastname', $lastname); $stmt->bindParam(':gender', $gender); $stmt->bindParam(':address', $address); $stmt->execute(); $conn = null; header('location: index.php'); }?>
update_student.php
<?php require_once 'conn.php'; if(ISSET($_POST['update'])){ $student_id = $_POST['student_id']; $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $gender = $_POST['gender']; $address = $_POST['address']; $query = "UPDATE `student` SET `firstname` = :firstname, `lastname` = :lastname, `gender` = :gender, `address` = :address WHERE `student_id` = :student_id"; $stmt = $conn->prepare($query); $stmt->bindParam(':firstname', $firstname); $stmt->bindParam(':lastname', $lastname); $stmt->bindParam(':gender', $gender); $stmt->bindParam(':address', $address); $stmt->bindParam(':student_id', $student_id); $stmt->execute(); $conn = null; header('location: index.php'); }?>
xóa.php
<?php require_once 'conn.php'; if(ISSET($_REQUEST['id'])){ $query = "DELETE FROM `student` WHERE student_id = '$_REQUEST[id]'"; $stmt = $conn->prepare($query); $stmt->execute(); $conn = null; header('location: index.php'); } ?>